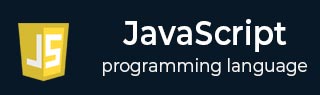
- Javascript Basics Tutorial
- Javascript - Home
- JavaScript - Overview
- JavaScript - Features
- JavaScript - Enabling
- JavaScript - Placement
- JavaScript - Syntax
- JavaScript - Hello World
- JavaScript - Console.log()
- JavaScript - Comments
- JavaScript - Variables
- JavaScript - let Statement
- JavaScript - Constants
- JavaScript - Data Types
- JavaScript - Type Conversions
- JavaScript - Strict Mode
- JavaScript - Reserved Keywords
- JavaScript Operators
- JavaScript - Operators
- JavaScript - Arithmetic Operators
- JavaScript - Comparison Operators
- JavaScript - Logical Operators
- JavaScript - Bitwise Operators
- JavaScript - Assignment Operators
- JavaScript - Conditional Operators
- JavaScript - typeof Operator
- JavaScript - Nullish Coalescing Operator
- JavaScript - Delete Operator
- JavaScript - Comma Operator
- JavaScript - Grouping Operator
- JavaScript - Yield Operator
- JavaScript - Spread Operator
- JavaScript - Exponentiation Operator
- JavaScript - Operator Precedence
- JavaScript Control Flow
- JavaScript - If...Else
- JavaScript - While Loop
- JavaScript - For Loop
- JavaScript - For...in
- Javascript - For...of
- JavaScript - Loop Control
- JavaScript - Break Statement
- JavaScript - Continue Statement
- JavaScript - Switch Case
- JavaScript - User Defined Iterators
- JavaScript Functions
- JavaScript - Functions
- JavaScript - Function Expressions
- JavaScript - Function Parameters
- JavaScript - Default Parameters
- JavaScript - Function() Constructor
- JavaScript - Function Hoisting
- JavaScript - Self-Invoking Functions
- JavaScript - Arrow Functions
- JavaScript - Function Invocation
- JavaScript - Function call()
- JavaScript - Function apply()
- JavaScript - Function bind()
- JavaScript - Closures
- JavaScript - Variable Scope
- JavaScript - Global Variables
- JavaScript - Smart Function Parameters
- JavaScript Objects
- JavaScript - Number
- JavaScript - Boolean
- JavaScript - Strings
- JavaScript - Arrays
- JavaScript - Date
- JavaScript - Math
- JavaScript - RegExp
- JavaScript - Symbol
- JavaScript - Sets
- JavaScript - WeakSet
- JavaScript - Maps
- JavaScript - WeakMap
- JavaScript - Iterables
- JavaScript - Reflect
- JavaScript - TypedArray
- JavaScript - Template Literals
- JavaScript - Tagged Templates
- Object Oriented JavaScript
- JavaScript - Objects
- JavaScript - Classes
- JavaScript - Object Properties
- JavaScript - Object Methods
- JavaScript - Static Methods
- JavaScript - Display Objects
- JavaScript - Object Accessors
- JavaScript - Object Constructors
- JavaScript - Native Prototypes
- JavaScript - ES5 Object Methods
- JavaScript - Encapsulation
- JavaScript - Inheritance
- JavaScript - Abstraction
- JavaScript - Polymorphism
- JavaScript - Destructuring Assignment
- JavaScript - Object Destructuring
- JavaScript - Array Destructuring
- JavaScript - Nested Destructuring
- JavaScript - Optional Chaining
- JavaScript - Global Object
- JavaScript - Mixins
- JavaScript - Proxies
- JavaScript Versions
- JavaScript - History
- JavaScript - Versions
- JavaScript - ES5
- JavaScript - ES6
- ECMAScript 2016
- ECMAScript 2017
- ECMAScript 2018
- ECMAScript 2019
- ECMAScript 2020
- ECMAScript 2021
- ECMAScript 2022
- JavaScript Cookies
- JavaScript - Cookies
- JavaScript - Cookie Attributes
- JavaScript - Deleting Cookies
- JavaScript Browser BOM
- JavaScript - Browser Object Model
- JavaScript - Window Object
- JavaScript - Document Object
- JavaScript - Screen Object
- JavaScript - History Object
- JavaScript - Navigator Object
- JavaScript - Location Object
- JavaScript - Console Object
- JavaScript Web APIs
- JavaScript - Web API
- JavaScript - History API
- JavaScript - Storage API
- JavaScript - Forms API
- JavaScript - Worker API
- JavaScript - Fetch API
- JavaScript - Geolocation API
- JavaScript Events
- JavaScript - Events
- JavaScript - DOM Events
- JavaScript - addEventListener()
- JavaScript - Mouse Events
- JavaScript - Keyboard Events
- JavaScript - Form Events
- JavaScript - Window/Document Events
- JavaScript - Event Delegation
- JavaScript - Event Bubbling
- JavaScript - Event Capturing
- JavaScript - Custom Events
- JavaScript Error Handling
- JavaScript - Error Handling
- JavaScript - try...catch
- JavaScript - Debugging
- JavaScript - Custom Errors
- JavaScript - Extending Errors
- JavaScript Important Keywords
- JavaScript - this Keyword
- JavaScript - void Keyword
- JavaScript - new Keyword
- JavaScript - var Keyword
- JavaScript HTML DOM
- JavaScript - HTML DOM
- JavaScript - DOM Methods
- JavaScript - DOM Document
- JavaScript - DOM Elements
- JavaScript - DOM Forms
- JavaScript - Changing HTML
- JavaScript - Changing CSS
- JavaScript - DOM Animation
- JavaScript - DOM Navigation
- JavaScript - DOM Collections
- JavaScript - DOM Node Lists
- JavaScript Miscellaneous
- JavaScript - Ajax
- JavaScript - Async Iteration
- JavaScript - Atomics Objects
- JavaScript - Rest Parameter
- JavaScript - Page Redirect
- JavaScript - Dialog Boxes
- JavaScript - Page Printing
- JavaScript - Validations
- JavaScript - Animation
- JavaScript - Multimedia
- JavaScript - Image Map
- JavaScript - Browsers
- JavaScript - JSON
- JavaScript - Multiline Strings
- JavaScript - Date Formats
- JavaScript - Get Date Methods
- JavaScript - Set Date Methods
- JavaScript - Modules
- JavaScript - Dynamic Imports
- JavaScript - BigInt
- JavaScript - Blob
- JavaScript - Unicode
- JavaScript - Shallow Copy
- JavaScript - Call Stack
- JavaScript - Reference Type
- JavaScript - IndexedDB
- JavaScript - Clickjacking Attack
- JavaScript - Currying
- JavaScript - Graphics
- JavaScript - Canvas
- JavaScript - Debouncing
- JavaScript - Performance
- JavaScript - Style Guide
- JavaScript Useful Resources
- JavaScript - Questions And Answers
- JavaScript - Quick Guide
- JavaScript - Functions
- JavaScript - Resources
JavaScript Number POSITIVE_INFINITY Property
The JavaScript Number POSITIVE_INFINITY is a static data property that represents the positive infinity value. The positive infinity value in JavaScript is the same as the positive value of the global "Infinity" property.
Note: If you try to access it using x.POSITIVE_INFINITY, where 'x', is a variable, it will return undefined.
Syntax
Following is the syntax of JavaScript Number POSITIVE_INFINITY property −
Number.POSITIVE_INFINITY
Parameters
- It does not accept any parameters.
Return value
This property has no return value.
Example 1
The following program demonstrates the usage of the JavaScript Number POSITIVE_INFINITY property. It will return 'Infinity' for Number.POSITIVE_INFINITY.
<html> <head> <title>JavaScript Number POSITIVE_INFINITY Property</title> </head> <body> <script> document.write("positive infinity = ", Number.POSITIVE_INFINITY); </script> </body> </html>
Output
The above program returns the positive infinity in JavaScript as 'infinity'.
positive infinity = Infinity
Example 2
If you try to access this property using any variable, it returns undefined.
The following is another example of the JavaScript Number POSITIVE_INFINITY property. Here, we are trying to find the positive infinity by using x.POSITIVE_INFINITY, where "x" is a variable with the value 2.
<html> <head> <title>JavaScript Number POSITIVE_INFINITY Property</title> </head> <body> <script> let x = 2; document.write("x = ", x); document.write("<br>positive infinity = ", x.POSITIVE_INFINITY); </script> </body> </html>
Output
This will return 'undefined' for x.POSITIVE_INFINITY.
x = 2 positive infinity = undefined
Example 3
If you multiply the Number.POSITIVE_INFINITY property with zero, the result will be NaN (Not a Number).
<html> <head> <title>JavaScript Number POSITIVE_INFINITY Property</title> </head> <body> <script> document.write("Result of 'Number.POSITIVE_INFINITY * 0' = ", Number.POSITIVE_INFINITY * 0); </script> </body> </html>
Output
The above program returns 'NaN' in the output −
Result of 'Number.POSITIVE_INFINITY * 0' = NaN
Example 4
In this example, we use the Number.POSITIVE_INFINITY property to check if a number is equal to positive infinity. If it is, we return a statement; otherwise, we return the number itself.
<html> <head> <title>JavaScript Number POSITIVE_INFINITY Property</title> </head> <body> <script> function check(num){ if(num == Number.POSITIVE_INFINITY){ return "Number is equal to positive infinity...!"; } else{ return num; } } //call the function document.write("Result of check(-Number.MAX_VALUE) is: ", check(-Number.MAX_VALUE)); document.write("<br>Result of check(-Number.MAX_VALUE * 2) is: ", check(-Number.MAX_VALUE*2)); </script> </body> </html>
Output
The above program returns output based on the satisfied condition −
Result of check(-Number.MAX_VALUE) is: -1.7976931348623157e+308 Result of check(-Number.MAX_VALUE * 2) is: Number is equal to positive infinity...!
To Continue Learning Please Login