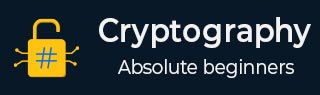
- Cryptography Tutorial
- Cryptography - Home
- Cryptography - Origin
- Cryptography - History
- Cryptography - Principles
- Cryptography - Applications
- Cryptography - Benefits & Drawbacks
- Cryptography - Modern Age
- Cryptography - Traditional Ciphers
- Cryptography - Need for Encryption
- Cryptography - Double Strength Encryption
- Cryptosystems
- Cryptosystems
- Cryptosystems - Components
- Attacks On Cryptosystem
- Cryptosystems - Rainbow table attack
- Cryptosystems - Dictionary attack
- Cryptosystems - Brute force attack
- Cryptosystems - Cryptanalysis Techniques
- Types of Cryptography
- Cryptosystems - Types
- Public Key Encryption
- Modern Symmetric Key Encryption
- Cryptography Hash functions
- Key Management
- Cryptosystems - Key Generation
- Cryptosystems - Key Storage
- Cryptosystems - Key Distribution
- Cryptosystems - Key Revocation
- Block Ciphers
- Cryptosystems - Stream Cipher
- Cryptography - Block Cipher
- Cryptography - Feistel Block Cipher
- Block Cipher Modes of Operation
- Block Cipher Modes of Operation
- Electronic Code Book (ECB) Mode
- Cipher Block Chaining (CBC) Mode
- Cipher Feedback (CFB) Mode
- Output Feedback (OFB) Mode
- Counter (CTR) Mode
- Classic Ciphers
- Cryptography - Reverse Cipher
- Cryptography - Caesar Cipher
- Cryptography - ROT13 Algorithm
- Cryptography - Transposition Cipher
- Cryptography - Encryption Transposition Cipher
- Cryptography - Decryption Transposition Cipher
- Cryptography - Multiplicative Cipher
- Cryptography - Affine Ciphers
- Cryptography - Simple Substitution Cipher
- Cryptography - Encryption of Simple Substitution Cipher
- Cryptography - Decryption of Simple Substitution Cipher
- Cryptography - Vigenere Cipher
- Cryptography - Implementing Vigenere Cipher
- Modern Ciphers
- Base64 Encoding & Decoding
- Cryptography - XOR Encryption
- Substitution techniques
- Cryptography - MonoAlphabetic Cipher
- Cryptography - Hacking Monoalphabetic Cipher
- Cryptography - Polyalphabetic Cipher
- Cryptography - Playfair Cipher
- Cryptography - Hill Cipher
- Polyalphabetic Ciphers
- Cryptography - One-Time Pad Cipher
- Implementation of One Time Pad Cipher
- Cryptography - Transposition Techniques
- Cryptography - Rail Fence Cipher
- Cryptography - Columnar Transposition
- Cryptography - Steganography
- Symmetric Algorithms
- Cryptography - Data Encryption
- Cryptography - Encryption Algorithms
- Cryptography - Data Encryption Standard
- Cryptography - Triple DES
- Cryptography - Double DES
- Advanced Encryption Standard
- Cryptography - AES Structure
- Cryptography - AES Transformation Function
- Cryptography - Substitute Bytes Transformation
- Cryptography - ShiftRows Transformation
- Cryptography - MixColumns Transformation
- Cryptography - AddRoundKey Transformation
- Cryptography - AES Key Expansion Algorithm
- Cryptography - Blowfish Algorithm
- Cryptography - SHA Algorithm
- Cryptography - RC4 Algorithm
- Cryptography - Camellia Encryption Algorithm
- Cryptography - ChaCha20 Encryption Algorithm
- Cryptography - CAST5 Encryption Algorithm
- Cryptography - SEED Encryption Algorithm
- Cryptography - SM4 Encryption Algorithm
- IDEA - International Data Encryption Algorithm
- Public Key (Asymmetric) Cryptography Algorithms
- Cryptography - RSA Algorithm
- Cryptography - RSA Encryption
- Cryptography - RSA Decryption
- Cryptography - Creating RSA Keys
- Cryptography - Hacking RSA Cipher
- Cryptography - ECDSA Algorithm
- Cryptography - DSA Algorithm
- Cryptography - Diffie-Hellman Algorithm
- Data Integrity in Cryptography
- Data Integrity in Cryptography
- Message Authentication
- Cryptography Digital signatures
- Public Key Infrastructure
- Cryptography Useful Resources
- Cryptography - Quick Guide
- Cryptography - Discussion
Cryptography - Base64 Encoding & Decoding
In this chapter, we will see the process of base64 encoding and decoding in Python, from the fundamentals to advanced approaches. So, let's get started with base64 encoding and decoding.
With the help of Base 64, an effective tool, we can transform binary data into a format that allows secure text transmission. So basically Base64 helps us for encoding and changing bytes into ASCII characters. It is useful when you want to send or use data in systems that only handle text. This encoding helps to ensure that the data remains secure and unchanged during transfer.
Details of Base64 encoding
Base64 refers to a group of related encoding techniques that encode binary data numerically and translate it into a base-64 representation. The word Base64 comes from a specific MIME-content transfer encoding.
Design
The specific characters used for making up the 64 characters required for Base64 vary for each implementation. The general rule is to select a set of 64 characters that are 1. part of a subset shared by most encodings, and 2. printable.
This combination makes it unlikely that the data will be modified while in transit through systems like email, which were previously not 8-bit clean. For example, the first 62 values in MIME's Base64 implementation are A-Z, a-z, and 0-9, with the final two being "+" and "/". Other versions, typically derived from Base64, share this attribute but differ in the symbols used for the last two values; for example, the URL and filename safe "RFC 4648 / Base64URL" variant uses "-" and "_".
Base64 has a specific set of characters −
26 Uppercase letters
26 Lowercase letters
10 Numbers
Plus sign (+) and slash (/) for new lines
It is commonly used in a variety of applications, including email attachments, data transmission via the internet, and storing complex data in simple text formats.
Base64 Module of Python
The Base64 module in Python provides functions for encoding and decoding data with the help of the Base64 encoding technique. This encoding strategy turns binary data into text format.
Below is an overview of the important functions of Base64 −
Key Functions of Base64
Sr.No. | Function & Description |
---|---|
1 | base64.b64encode(s, altchars=None) This function encodes the input bytes-like object s using Base64 and returns the encoded bytes. To generate URL or file system safe Base64 strings, you can supply an alternate set of characters (altchars) to substitute the standard '+' and '/' characters. |
2 | base64.b64decode(s, altchars=None, validate=False) Decodes the Base64 encoded bytes-like object or ASCII string s and returns the decoded bytes. Similar to the b64encode function, you can specify an alternative set of characters (altchars). If characters outside of the Base64 alphabet are present in the input, you can enable validation (validate=True) to report an error. |
3 | base64.standard_b64encode(s) This function encodes the input bytes-like object s using the standard Base64 alphabet and returns the encoded bytes. |
4 | base64.standard_b64decode(s) This function decodes the bytes-like object or ASCII string s using the standard Base64 alphabet and returns the decoded bytes. |
5 | base64.urlsafe_b64encode(s) This function encodes the input bytes-like object s using a URL- and filesystem-safe alphabet, which substitutes '-' instead of '+' and '_' instead of '/' in the standard Base64 alphabet. The result may still contain '='. |
6 | base64.urlsafe_b64decode(s) This function decodes the bytes-like object or ASCII string s using the URL- and filesystem-safe alphabet and returns the decoded bytes. |
Implementation using Python
Here is how we can do Base64 encoding and decoding in Python −
Encoding using Base64
Encoding is the method in which we transform our plaintext in an encrypted form so that the middle person can not understand it. So here i am providing two different methods for encoding using Base64 in Python −
Using the base64.b64encode() function
In this code, we will explore how to use Python's base64 module to encode data in a format that can be transmitted or stored in text-based systems. To encode the message, we will use the method base64.b64encode(). This function converts the data into a format that is safe for transmission over systems that handle only text. It is a simple example for understanding the concept of Base64 encoding.
Below is the implementation of Base64 encoding using the above function −
Example
import base64 def base64_encoding(data): encoded_bytes = base64.b64encode(data) encoded_string = encoded_bytes.decode('utf-8') return encoded_string #our plain text message data = b"Hello, World!" encoded_data = base64_encoding(data) print("Our plain text message:", data) print("Encoded data using b64encode():", encoded_data)
Following is the output of the above example −
Input/Output
Our plain text message: b'Hello, World!' Encoded data using b64encode(): SGVsbG8sIFdvcmxkIQ==
Using the base64.standard_b64encode() function
The code imports the base64 module, which contains functions for encoding and decoding data using the Base64 encoding method. The function encodes the input data with the help of the base64.standard_b64encode() method. This function converts the data into a Base64-encoded format using the standard Base64 alphabet.
Below is the Python implementation using standard_b64encode() function −
Example
import base64 def base64_encoding(data): encoded_bytes = base64.standard_b64encode(data) encoded_string = encoded_bytes.decode('utf-8') return encoded_string # Our plaintext example data = b"Hello, Tutorialspoint!" encoded_data = base64_encoding(data) print("Our plain text message:", data) print("Encoded data using standard_b64encode() :", encoded_data)
Following is the output of the above example −
Input/Output
Our plain text message: b'Hello, Tutorialspoint!' Encoded data using standard_b64encode() : SGVsbG8sIFR1dG9yaWFsc3BvaW50IQ==
Using urlsafe_b64encode()
In this example, we will use the urlsafe_b64encode() method to return a base64 encoded text designed to use in URLs. This is useful when we need to add binary data in a URL, like a path segment or a query string.
Here is the implementation of Base64 encoding using the above function −
Example
import base64 data = b'Hello, Bharat!' encoded_data = base64.urlsafe_b64encode(data) print("Our plaintext message: ", data) print("Encoded data using urlsafe_b64encode(): ", encoded_data)
Following is the output of the above example −
Input/Output
Our plaintext message: b'Hello, Bharat!' Encoded data using urlsafe_b64encode(): b'SGVsbG8sIEJoYXJhdCE='
Decoding using Base64
Decoding is the way of converting encoded or encrypted data back into its original, human-readable format.
Here are two Python programs, the first program will decode a Base64-encoded string with the help of the base64.b64decode() function, and another will use the base64.standard_b64decode() function.
Using base64.b64decode()
The program achieves the same result but uses different functions from the base64 module for decoding. IT directly uses base64.b64decode() to decode the Base64-encoded string.
Following is the Python implementation for the simple decoding using b64decode() function −
Example
import base64 def base64_decoding(encoded_string): decoded_bytes = base64.b64decode(encoded_string) decoded_string = decoded_bytes.decode('utf-8') return decoded_string # Our ciphertext encoded_data = "SGVsbG8sIFR1dG9yaWFsc3BvaW50IQ==" decoded_data = base64_decoding(encoded_data) print("Our encoded message:", encoded_data) print("Decoded data using b64decode():", decoded_data)
Following is the output of the above example −
Input/Output
Our encoded message: SGVsbG8sIFR1dG9yaWFsc3BvaW50IQ== Decoded data using b64decode(): Hello, Tutorialspoint!
Using base64.standard_b64decode()
This program uses the base64.standard_b64decode() function to decode the encrypted message. So here is a Python implementation for the Base64 decoding −
Example
import base64 def base64_decoding(encoded_string): decoded_bytes = base64.standard_b64decode(encoded_string) decoded_string = decoded_bytes.decode('utf-8') return decoded_string # our encrypted data encoded_data = "SGVsbG8sIEV2ZXJ5b25lIQ==" decoded_data = base64_decoding(encoded_data) print("Our encoded message:", encoded_data) print("Decoded data using standard_b64decode():", decoded_data)
Following is the output of the above example −
Input/Output
Our encoded message: SGVsbG8sIEV2ZXJ5b25lIQ== Decoded data using standard_b64decode(): Hello, Everyone!
Handle Different Data Types using Base64
When we go deep in Base64 encoding we may face some situations in which we need to encode data types other than simple text messages. So we will see how we can encode an image and JSON data using Base64 functions.
Encode an Image file
Let's see an example in which we will have an image file so we will encode it in base64. When we open the image file in binary mode using the open() function. So we will read the content of the image file with the help of the read() method. And then we will use the b64encode() function to encode the binary data of the image file. The output will be a base64 encoded byte string showing the image data.
Here is how we can do it −
Example
import base64 # Binary data (image) with open('image.jpg', 'rb') as file: binary_data = file.read() # Encode binary data encoded_binary_data = base64.b64encode(binary_data).decode('utf-8') print("Encoded (Image) Binary Data:", encoded_binary_data)
In this example you can replace the image file path with your image file name and path.
Following is the output of the above example −
Input/Output
The code for base64 encoding gives you the following output −

Encoding the JSON Object
One more basic use case Base64 is encoding a JSON object. So in this we will first define a JSON object. And then we will convert the JSON object to a string with the help of the dumps() function from the JSON module. This string is then converted to bytes and passed to the b64encode() function to be encoded.
Here is the implementation −
Example
import base64 import json # Declare a JSON object here json_obj = { 'name': 'Amit Sharma', 'age': 28, 'city': 'New Delhi' } # change the JSON object to a string json_string = json.dumps(json_obj) # change the string to bytes byte_data = json_string.encode('utf-8') # Encode the bytes encoded_data = base64.b64encode(byte_data) print("Encoded JSON Object: ", encoded_data)
Following is the output of the above example −
Input/Output
Encoded JSON Object: b'eyJuYW1lIjogIkFtaXQgU2hhcm1hIiwgImFnZSI6IDI4LCAiY2l0eSI6ICJOZXcgRGVsaGkifQ=='
As you can see, base64 encoding in Python is very flexible and can handle a wide range of data formats. Python's base64 module can encode texts, image files, and JSON objects.
Applications
Base64 encoding is often used in a variety of applications. It is widely used to encrypt binary data, especially if it needs to be communicated by email or used in other text fields. It is also used in a variety of web and internet protocols, as well as to encode digital signatures and certificates.
Limitations
Here are the limitations of Base64 encoding −
Base64 encoding usually maximizes the size of the data by about 33%. This can impact transmission and storage efficiency, mainly for large datasets.
It is basically not a form of encryption. It simply converts data into a different format. As a result, sensitive information is not kept confidential or secure.
Base64 adds padding characters ('=') to the end of the encoded data to ensure it aligns properly. This way it can complicate parsing and processing of the encoded data.
It uses a limited set of characters (A-Z, a-z, 0-9, '+', '/') for encoding. This can lead to issues when the encoded data needs to be transmitted or processed in systems that have restrictions on certain characters.
The encoding of Base64 does not compress data. It is only meant for representing binary data in a text-based format, not for reducing file size.
Difference between ASCII and base64
You can observe the following differences when you work on ASCII and base64 for encoding data −
When you encode text in ASCII, you start with a text string and convert it to a sequence of bytes.
When you encode data in Base64, you start with a sequence of bytes and convert it to a text string.
Drawback
Base64 algorithm is usually used to store passwords in database. The major drawback is that each decoded word can be encoded easily through any online tool and intruders can easily get the information.
To Continue Learning Please Login