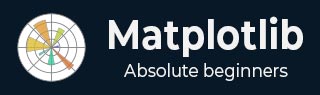
- Matplotlib Basics
- Matplotlib - Home
- Matplotlib - Introduction
- Matplotlib - Vs Seaborn
- Matplotlib - Environment Setup
- Matplotlib - Anaconda distribution
- Matplotlib - Jupyter Notebook
- Matplotlib - Pyplot API
- Matplotlib - Simple Plot
- Matplotlib - Saving Figures
- Matplotlib - Markers
- Matplotlib - Figures
- Matplotlib - Styles
- Matplotlib - Legends
- Matplotlib - Colors
- Matplotlib - Colormaps
- Matplotlib - Colormap Normalization
- Matplotlib - Choosing Colormaps
- Matplotlib - Colorbars
- Matplotlib - Text
- Matplotlib - Text properties
- Matplotlib - Subplot Titles
- Matplotlib - Images
- Matplotlib - Image Masking
- Matplotlib - Annotations
- Matplotlib - Arrows
- Matplotlib - Fonts
- Matplotlib - What are Fonts?
- Setting Font Properties Globally
- Matplotlib - Font Indexing
- Matplotlib - Font Properties
- Matplotlib - Scales
- Matplotlib - Linear and Logarthmic Scales
- Matplotlib - Symmetrical Logarithmic and Logit Scales
- Matplotlib - LaTeX
- Matplotlib - What is LaTeX?
- Matplotlib - LaTeX for Mathematical Expressions
- Matplotlib - LaTeX Text Formatting in Annotations
- Matplotlib - PostScript
- Enabling LaTex Rendering in Annotations
- Matplotlib - Mathematical Expressions
- Matplotlib - Animations
- Matplotlib - Artists
- Matplotlib - Styling with Cycler
- Matplotlib - Paths
- Matplotlib - Path Effects
- Matplotlib - Transforms
- Matplotlib - Ticks and Tick Labels
- Matplotlib - Radian Ticks
- Matplotlib - Dateticks
- Matplotlib - Tick Formatters
- Matplotlib - Tick Locators
- Matplotlib - Basic Units
- Matplotlib - Autoscaling
- Matplotlib - Reverse Axes
- Matplotlib - Logarithmic Axes
- Matplotlib - Symlog
- Matplotlib - Unit Handling
- Matplotlib - Ellipse with Units
- Matplotlib - Spines
- Matplotlib - Axis Ranges
- Matplotlib - Axis Scales
- Matplotlib - Axis Ticks
- Matplotlib - Formatting Axes
- Matplotlib - Axes Class
- Matplotlib - Twin Axes
- Matplotlib - Figure Class
- Matplotlib - Multiplots
- Matplotlib - Grids
- Matplotlib - Object-oriented Interface
- Matplotlib - PyLab module
- Matplotlib - Subplots() Function
- Matplotlib - Subplot2grid() Function
- Matplotlib - Anchored Artists
- Matplotlib - Manual Contour
- Matplotlib - Coords Report
- Matplotlib - AGG filter
- Matplotlib - Ribbon Box
- Matplotlib - Fill Spiral
- Matplotlib - Findobj Demo
- Matplotlib - Hyperlinks
- Matplotlib - Image Thumbnail
- Matplotlib - Plotting with Keywords
- Matplotlib - Create Logo
- Matplotlib - Multipage PDF
- Matplotlib - Multiprocessing
- Matplotlib - Print Stdout
- Matplotlib - Compound Path
- Matplotlib - Sankey Class
- Matplotlib - MRI with EEG
- Matplotlib - Stylesheets
- Matplotlib - Background Colors
- Matplotlib - Basemap
- Matplotlib Event Handling
- Matplotlib - Event Handling
- Matplotlib - Close Event
- Matplotlib - Mouse Move
- Matplotlib - Click Events
- Matplotlib - Scroll Event
- Matplotlib - Keypress Event
- Matplotlib - Pick Event
- Matplotlib - Looking Glass
- Matplotlib - Path Editor
- Matplotlib - Poly Editor
- Matplotlib - Timers
- Matplotlib - Viewlims
- Matplotlib - Zoom Window
- Matplotlib Plotting
- Matplotlib - Bar Graphs
- Matplotlib - Histogram
- Matplotlib - Pie Chart
- Matplotlib - Scatter Plot
- Matplotlib - Box Plot
- Matplotlib - Violin Plot
- Matplotlib - Contour Plot
- Matplotlib - 3D Plotting
- Matplotlib - 3D Contours
- Matplotlib - 3D Wireframe Plot
- Matplotlib - 3D Surface Plot
- Matplotlib - Quiver Plot
- Matplotlib Useful Resources
- Matplotlib - Quick Guide
- Matplotlib - Useful Resources
- Matplotlib - Discussion
Matplotlib - Transforms
Transform in general is a way to change something from one form to another. It is like taking an input and turning it into a different output. Transforms are used in various fields, such as mathematics, physics, and computer science.
For example, in mathematics, a transform can be a mathematical operation that changes a set of numbers or functions into a different representation. The Fourier transform, for instance, converts a function in the time domain (like a sound wave) into its frequency components.

Transforms in Matplotlib
In Matplotlib, transforms refer to the conversion process from data coordinates to pixel coordinates, allowing to place the graphical elements such as points, lines, and text within a plot accurately.
You can use various types of transformations in matplotlib, such as linear transformations, logarithmic transformations, and more. To handle these transformations, Matplotlib provides the "transform" parameter.
The "transform" parameter can take various values, such as instances of the "matplotlib.transforms.Transform" class or strings representing common transformations like 'ax.transData' for data coordinates or 'ax.transAxes' for axes coordinates. By using the transform parameter, you can customize the coordinate system and apply different transformations to enhance the visualization of their data on the Matplotlib plots.
Data to Axes Transform
In Matplotlib, the data to axes transform refers to the process of converting your actual data points into coordinates within the axes of your plot. When you plot data, you use specific "x" and "y" values. The transformation ensures that these data points are correctly positioned within the axes of your plot, taking into account the scaling and limits of the "x" and "y" axes.
Example
In the following example, we are creating a simple line plot using data coordinates (x, y). We then use the ax.text() function to add text to the plot, specifying the position (2, 25) in data coordinates. The "transform=ax.transData" parameter ensures that the text is positioned using data coordinates −
import matplotlib.pyplot as plt # Data x = [1, 2, 3, 4] y = [10, 20, 25, 30] # Creating a plot fig, ax = plt.subplots() ax.plot(x, y, marker='o', linestyle='-', label='Data points') ax.legend() # Transforming data coordinates to axes coordinates ax.text(2, 25,'Text in Data Coordinates' , transform=ax.transData) # Setting plot title and labels ax.set_title('Data to Axes Transform') ax.set_xlabel('X-axis') ax.set_ylabel('Y-axis') plt.show()
Output
Following is the output of the above code −

Axes to Data Transform
The axes to data transform in Matplotlib is the reverse of the "data to axes transform". While the "data to axes transform" converts your actual data points into coordinates within the axes of your plot, the "axes to data transform" does the opposite. It takes coordinates specified in terms of the axes of your plot and converts them into the corresponding data values.
Example
In here, we are creating a plot with axis limits set between "0" and "1" for both "x" and "y" axes. We use the ax.text() function to add text to the plot, specifying the position "(0.5, 0.5)" in axes coordinates. The "transform=ax.transAxes" parameter ensures that the text position is interpreted as relative to the axes rather than the data −
import matplotlib.pyplot as plt # Creating a plot with normalized axes fig, ax = plt.subplots() ax.set_xlim(0, 1) ax.set_ylim(0, 1) # Transforming axes coordinates to data coordinates ax.text(0.5, 0.5, 'Text in Axes Coordinates', transform=ax.transAxes, ha='center', va='center') # Setting plot title and labels ax.set_title('Axes to Data Transform Example') ax.set_xlabel('X-axis (normalized)') ax.set_ylabel('Y-axis (normalized)') plt.show()
Output
On executing the above code we will get the following output −

Blended Transform
In Matplotlib, a blended transform is a way to combine or blend different coordinate systems when placing elements on a plot. It allows you to create a custom transformation that contains aspects of both data coordinates and axes coordinates.
Example
In the example below, we are creating a plot and using the blended_transform_factory() function to create a blended transformation. The resulting "trans" object is a blend of both data and axes coordinates. The ax.text() function then uses this blended transformation to position text at (0.8, 0.2), effectively combining data and axes coordinates −
import matplotlib.pyplot as plt from matplotlib.transforms import blended_transform_factory # Creating a plot fig, ax = plt.subplots() # Creating a blended transformation trans = blended_transform_factory(ax.transData, ax.transAxes) # Adding text using the blended transformation ax.text(0.8, 0.2, 'Blended Transform', transform=trans, ha='center', va='center') # Setting plot title and labels ax.set_title('Blended Transform') ax.set_xlabel('X-axis') ax.set_ylabel('Y-axis') plt.show()
Output
After executing the above code, we get the following output −

Offset Transform
In Matplotlib, an offset transform is a way to add a precise offset to the position of elements on a plot. It involves shifting or translating the coordinates of the elements by a specified amount. This type of transformation is useful when you want to fine-tune the placement of text, markers, or other graphical elements.
For example, if you have a data point at (2, 10) and you want to display a label slightly to the right and above this point, you can use an Offset Transform to apply a specific offset to the original coordinates. This allows you to control the exact positioning of elements relative to their original locations in the data.
Example
Now, we are creating a plot, and using the "ScaledTranslation" class to create an offset transformation. The "trans" object is a combination of the original data transformation (ax.transData) and a scaled translation of (0.1, 0.2) in data coordinates. The ax.text() function then uses this transformed coordinate to position text at (2, 25) with the specified offset −
import matplotlib.pyplot as plt from matplotlib.transforms import ScaledTranslation # Creating a plot fig, ax = plt.subplots() # Creating an offset transformation trans = ax.transData + ScaledTranslation(0.1, 0.2, ax.transData) # Adding text with the offset transformation ax.text(0.4, 0.2, 'Text with Offset', transform=trans) # Setting plot title and labels ax.set_title('Offset Transform Example') ax.set_xlabel('X-axis') ax.set_ylabel('Y-axis') plt.show()
Output
On executing the above code we will get the following output −

To Continue Learning Please Login