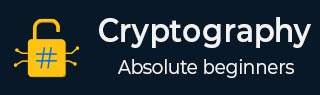
- Cryptography Tutorial
- Cryptography - Home
- Cryptography - Origin
- Cryptography - History
- Cryptography - Principles
- Cryptography - Applications
- Cryptography - Benefits & Drawbacks
- Cryptography - Modern Age
- Cryptography - Traditional Ciphers
- Cryptography - Need for Encryption
- Cryptography - Double Strength Encryption
- Cryptosystems
- Cryptosystems
- Cryptosystems - Components
- Attacks On Cryptosystem
- Cryptosystems - Rainbow table attack
- Cryptosystems - Dictionary attack
- Cryptosystems - Brute force attack
- Cryptosystems - Cryptanalysis Techniques
- Types of Cryptography
- Cryptosystems - Types
- Public Key Encryption
- Modern Symmetric Key Encryption
- Cryptography Hash functions
- Key Management
- Cryptosystems - Key Generation
- Cryptosystems - Key Storage
- Cryptosystems - Key Distribution
- Cryptosystems - Key Revocation
- Block Ciphers
- Cryptosystems - Stream Cipher
- Cryptography - Block Cipher
- Cryptography - Feistel Block Cipher
- Block Cipher Modes of Operation
- Electronic Code Book (ECB) Mode
- Cipher Block Chaining (CBC) Mode
- Cipher Feedback (CFB) Mode
- Output Feedback (OFB) Mode
- Counter (CTR) Mode
- Classic Ciphers
- Cryptography - Reverse Cipher
- Cryptography - Caesar Cipher
- Cryptography - ROT13 Algorithm
- Cryptography - Transposition Cipher
- Cryptography - Encryption Transposition Cipher
- Cryptography - Decryption Transposition Cipher
- Cryptography - Multiplicative Cipher
- Cryptography - Affine Ciphers
- Cryptography - Simple Substitution Cipher
- Cryptography - Encryption of Simple Substitution Cipher
- Cryptography - Decryption of Simple Substitution Cipher
- Cryptography - Vigenere Cipher
- Cryptography - Implementing Vigenere Cipher
- Modern Ciphers
- Base64 Encoding & Decoding
- Cryptography - XOR Encryption
- Substitution techniques
- Cryptography - MonoAlphabetic Cipher
- Cryptography - Hacking Monoalphabetic Cipher
- Cryptography - Polyalphabetic Cipher
- Cryptography - Playfair Cipher
- Cryptography - Hill Cipher
- Polyalphabetic Ciphers
- Cryptography - One-Time Pad Cipher
- Implementation of One Time Pad Cipher
- Cryptography - Transposition Techniques
- Cryptography - Rail Fence Cipher
- Cryptography - Columnar Transposition
- Cryptography - Steganography
- Symmetric Algorithms
- Cryptography - Data Encryption
- Cryptography - Encryption Algorithms
- Cryptography - Data Encryption Standard
- Cryptography - Triple DES
- Cryptography - Double DES
- Advanced Encryption Standard
- Cryptography - AES Structure
- Cryptography - AES Transformation Function
- Cryptography - Substitute Bytes Transformation
- Data Integrity in Cryptography
- Data Integrity in Cryptography
- Message Authentication
- Cryptography Digital signatures
- Public Key Infrastructure
- Cryptography Useful Resources
- Cryptography - Quick Guide
- Cryptography - Discussion
Cryptography - Multiplicative Cipher
In this chapter we will deep dive into the Multiplicative Cipher Algorithm and how it works! So let's see it in the sections below.
A multiplicative cipher belongs to the category of monoalphabetic ciphers. In a multiplicative cipher, every letter in the plaintext is substituted with a matching letter from the ciphertext based on a predetermined multiplication key.
The main idea behind the multiplicative cipher is to encode and decode the plaintext using the modular arithmetic of the integers modulo, a large prime number used as a multiplication key. Each letter's numerical value in the plaintext is multiplied by the key all over the encryption process, and the result is then calculated modulo the key. For example, if the plaintext letter is "s" and the key is 7, then the ciphertext letter is (18*7) mod 26 = 22 since the numerical value of "s" is 18. So, in this case, the cipher text symbol for the letter "a" will be "w."
Decryption is just the encryption process done backwards: each letter in the ciphertext is given a numerical value, which is divided by the key, and the result is then taken modulo the key. The multiplicative cipher's simplicity−that is, its ease of implementation, understanding, and low processing power requirements−is one of its primary benefits.
But this method is easy to use and understand, it is not very secure. It is similar to a secret code that is simple for someone else to decipher. For small things, it is suitable, but not for important things that require strong protection.
How does Multiplicative Cipher work?
Your secret key is a big prime number that you choose. You then modify each letter in your message using mathematical operations. For example, if your key is 7 and your message has the letter 's', which is number 18, you do (18 times 7) divided by 26 and take the remainder. This gives you 22, which corresponds to the letter 'w'. So, 's' becomes 'w' in the secret message.
All you have to do is reverse the math to read the secret message. You can divide each letter's number with the key and get the remainder.
If multiplication is used to convert to cipher text, it is called a wrap-around situation. Consider the letters and the associated numbers to be used as shown below −
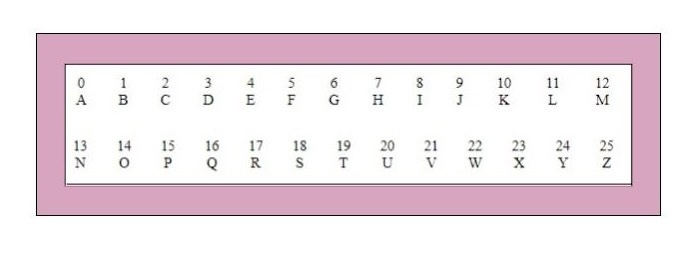
The numbers are what will be multiplied, and the corresponding key is 7. To create a multiplicative cipher in such a situation, the fundamental formula is as follows −
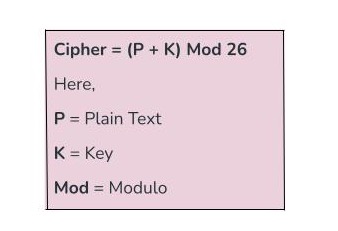
Now use the above formula to convert "hello" in cipher text using a key, K. Let us assume our key, K is 3. Here is how we will apply the given formula to each letter in the word "hello" −
For 'h': P (plaintext value of 'h') = 7 (assuming 'a' is 0, 'b' is 1, ..., 'h' is 7)
Cipher = (7 + 3) Mod 26 = 10 Mod 26 = 10
For 'e': P (plaintext value of 'e') = 4
Cipher = (4 + 3) Mod 26 = 7 Mod 26 = 7
For 'l': P (plaintext value of 'l') = 11
Cipher = (11 + 3) Mod 26 = 14 Mod 26 = 14
For 'l': P (plaintext value of 'l') = 11
Cipher = (11 + 3) Mod 26 = 14 Mod 26 = 14
For 'o': P (plaintext value of 'o') = 14
Cipher = (14 + 3) Mod 26 = 17 Mod 26 = 17
So values regarding the numbers 10, 7, 14, 14, 17 are k, h, o, o, r respectively.
So, the ciphertext for "hello" with a key of 3 will be "khoor".
Algorithms for Multiplicative Cipher
Encryption Algorithm
First you need to choose a prime number as the encryption key.
Assign numerical values to each letter in the plaintext message.
Multiply each numerical value by the encryption key.
Take the result modulo 26 which is the number of letters in the English alphabet to make sure that the result should stay within the range of the alphabet.
Convert the resulting numerical values back to letters to get the ciphertext message.
Decryption Algorithm
Choose the same prime number as the decryption key.
Assign numerical values to each letter in the ciphertext message.
Divide each numerical value by the decryption key.
Take the result modulo 26.
Convert the resulting numerical values back to letters to get the original plaintext message.
Implementation using Python
So we can implement this algorithm in different ways −
Using Basic Arithmetics
In this example we are going to use basic arithmetic operations for encryption and decryption in the multiplicative cipher. So in the encryption process we will convert the plaintext message to numbers (0-25) using ASCII values. Each number will be multiplied by the encryption key. So the result will be taken modulo 26 to ensure it falls within the range of the alphabet (0-25). And then we will convert the numbers back to characters using ASCII values.
In the decryption process we will convert the ciphertext message to numbers (0-25) using ASCII values. Each number is multiplied by the modular multiplicative inverse of the encryption key. The result is then taken modulo 26. And then resulting numbers are converted back to characters using ASCII values.
Example
Below is a simple Python code for the multiplicative cipher algorithm. See the program below −
def multiplicative_encrypt(message, key): cipher_text = '' for char in message: if char.isalpha(): # Change char to number (0-25) num = ord(char.lower()) - ord('a') # Encrypt with the help of (P * K) % 26 encrypted_num = (num * key) % 26 # change back to character cipher_text += chr(encrypted_num + ord('a')) else: cipher_text += char return cipher_text def multiplicative_decrypt(ciphertext, key): plain_text = '' # get the modular multiplicative inverse of the key inverse_key = pow(key, -1, 26) for char in ciphertext: if char.isalpha(): # change char to number (0-25) num = ord(char.lower()) - ord('a') # Decrypt using the formula (C * K_inverse) % 26 decrypted_num = (num * inverse_key) % 26 # change back to character plain_text += chr(decrypted_num + ord('a')) else: plain_text += char return plain_text plaintext = "hello tutorialspoint" key = 7 encrypted_message = multiplicative_encrypt(plaintext, key) print("Encrypted message:", encrypted_message) decrypted_message = multiplicative_decrypt(encrypted_message, key) print("Decrypted message:", decrypted_message)
Following is the output of the above example −
Input/Output
Encrypted message: xczzu dkdupeazwbuend Decrypted message: hello tutorialspoint
Using sympy and math library
In the below example we are going to use sympy and math libraries of Python for different purposes −
Sympy Library − From the sympy library we will import the mod_inverse function. This function will be used to calculate the modular multiplicative inverse of a number. In the multiplicative cipher, this function is used to find the multiplicative inverse of the encryption/decryption key modulo 26.
Math Library − So we will import the entire math library. And we will use the math.gcd() function from the math library to calculate the greatest common divisor (GCD) of 26 and the encryption/decryption key. The GCD is used to check if the key is coprime with 26. This check is important for making sure that the key is valid for encryption.
So simply we can say that the sympy library is used to calculate the modular multiplicative inverse, and the math library is used to calculate the greatest common divisor for key validation. Both libraries provide mathematical functions that are useful in implementing the multiplicative cipher algorithm.
Example
Following is a simple Python code for Multiplicative cipher algorithm. Please check the code below −
from sympy import mod_inverse import math def multiplicative_cipher(text, mode, key): char_to_num = {} result_message = '' # dictionary mapping characters to their numerical values for i in range(26): char_to_num[chr(ord('a') + i)] = i # List of characters char_list = list(char_to_num.keys()) # Dictionary mapping numerical values to characters num_to_char = dict(zip(char_to_num.values(), char_to_num.keys())) if mode == 'encrypt': if math.gcd(26, key) == 1: # Encryption for char in text: if char in char_list: # Encrypt each character new_num = (char_to_num[char] * key) % 26 result_message += num_to_char[new_num] else: # Leave non-alphabetic characters unchanged result_message += char else: print('Invalid key selected') elif mode == 'decrypt': # Calculate the multiplicative inverse of the key inv_key = mod_inverse(key, 26) # Decryption for char in text: if char in char_list: # Decrypt each character new_num = (char_to_num[char] * inv_key) % 26 result_message += num_to_char[new_num] else: # Leave non-alphabetic characters unchanged result_message += char return result_message encrypted_text = multiplicative_cipher('hello world', 'encrypt', 7) print("Encrypted message:", encrypted_text) decrypted_text = multiplicative_cipher('xczzu yupzv', 'decrypt', 7) print("Decrypted message:", decrypted_text)
Following is the output of the above example −
Input/Output
Encrypted message: xczzu yupzv Decrypted message: hello world
Implementation using Java
Now we will use Java programming language to create a program for multiplicative cipher. In which we will have a class, and two functions with the help of it we will encrypt and decrypt the given plaintext message.
Example
So the implementation using Java programming langugage is as follows −
public class MultiplicativeCipher { private int key; public MultiplicativeCipher(int k) { key = k; } public String encrypt(String plaintext) { StringBuilder ciphertext = new StringBuilder(); for (char c : plaintext.toCharArray()) { if (Character.isLetter(c)) { if (Character.isUpperCase(c)) ciphertext.append((char)(((c - 'A') * key) % 26 + 'A')); else ciphertext.append((char)(((c - 'a') * key) % 26 + 'a')); } else { ciphertext.append(c); } } return ciphertext.toString(); } public String decrypt(String ciphertext) { StringBuilder plaintext = new StringBuilder(); int modInverse = 0; for (int i = 0; i < 26; i++) { if ((key * i) % 26 == 1) { modInverse = i; break; } } for (char c : ciphertext.toCharArray()) { if (Character.isLetter(c)) { if (Character.isUpperCase(c)) plaintext.append((char)(((c - 'A') * modInverse) % 26 + 'A')); else plaintext.append((char)(((c - 'a') * modInverse) % 26 + 'a')); } else { plaintext.append(c); } } return plaintext.toString(); } public static void main(String[] args) { MultiplicativeCipher cipher = new MultiplicativeCipher(3); // Set the key to 3 String plaintext = "Hello world, How are you"; String ciphertext = cipher.encrypt(plaintext); System.out.println("The Encrypted text: " + ciphertext); System.out.println("The Decrypted text: " + cipher.decrypt(ciphertext)); } }
Following is the output of the above example −
Input/Output
The Encrypted text: Vmhhq oqzhj, Vqo azm uqi The Decrypted text: Hello world, How are you
Implementation using C++
We are going to use C++ programming langugage to implenent the multiplicative cipher. So we will have a class called MultiplicativeCipher. It offers tools for both message encrypting and decrypting. Using the supplied key, encrypt() method will encrypt a string of text input and returns the result. And using the given key, decrypt() method will decrypt a string of ciphertext and returns the plaintext.
Example
So the implementation of multiplicative cipher using C++ is as follows −
#include <iostream> #include <string> using namespace std; class MultiplicativeCipher { private: int key; public: MultiplicativeCipher(int k) { key = k; } string encrypt(string plaintext) { string ciphertext = ""; for (char& c : plaintext) { if (isalpha(c)) { if (isupper(c)) ciphertext += char(((c - 'A') * key) % 26 + 'A'); else ciphertext += char(((c - 'a') * key) % 26 + 'a'); } else { ciphertext += c; } } return ciphertext; } string decrypt(string ciphertext) { string plaintext = ""; int modInverse = 0; for (int i = 0; i < 26; i++) { if ((key * i) % 26 == 1) { modInverse = i; break; } } for (char& c : ciphertext) { if (isalpha(c)) { if (isupper(c)) plaintext += char(((c - 'A') * modInverse) % 26 + 'A'); else plaintext += char(((c - 'a') * modInverse) % 26 + 'a'); } else { plaintext += c; } } return plaintext; } }; int main() { MultiplicativeCipher cipher(3); // Set the key to 3 string plaintext = "hello this world is awesome!"; string ciphertext = cipher.encrypt(plaintext); cout << "The Encrypted text: " << ciphertext << endl; cout << "The Decrypted text: " << cipher.decrypt(ciphertext) << endl; return 0; }
Following is the output of the above example −
Input/Output
The Encrypted text: vmhhq fvyc oqzhj yc aomcqkm! The Decrypted text: hello this world is awesome!
Features of Multiplicative Cipher
Here are some main features of Multiplicative Cipher −
Multiplicative cipher is simple to understand and use. It follows a straightforward method of replacing letters in a message.
It relies on prime numbers as keys for encryption and decryption.
The cipher uses modular arithmetic, in which we take remainders after division. This method helps us to keep the encrypted values within a certain range, in the case of multiplicative cipher it is the size of the alphabet.
As it is very easy to implement, it is not secure for transmitting sensitive information. It can be easily cracked with basic techniques.
It does not need much computational power to encrypt or decrypt messages.
Multiplicative cipher is suitable for small-scale applications or scenarios where high security is not a concern.
Summary
In this chapter, we have learned about Multiplicative Cipher and its implementations. Multiplicative cipher is a way to change a message into secret code by replacing each letter with another letter using multiplication with a special number, called the key. There are different ways to implement this cipher technique and we have used different programming langugages like Python, C++ and Java. You can use basic arithmetic operations or libraries like sympy and math. The code examples show how to encrypt and decrypt messages using these methods. You can use them as per your understanding and usage.
To Continue Learning Please Login