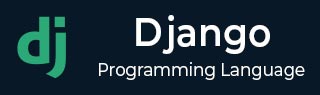
- Django Basic Concepts
- Django - Home
- Django - Basics
- Django - Overview
- Django - Environment
- Django - Creating a Project
- Django - Apps Life Cycle
- Django - Creating Views
- Django - URL Mapping
- Django - Templates System
- Django - MVT
- Django Admin
- Django Admin - Interface
- Django Admin - Create User
- Django Admin - Include Models
- Django Admin - Set Fields to Display
- Django Admin - Update Objects
- Django Models
- Django - Models
- Django - Insert Data
- Django - Update Data
- Django - Delete Data
- Django - Update Model
- Django Advanced
- Django - Page Redirection
- Django - Sending E-mails
- Django - Generic Views
- Django - Form Processing
- Django - File Uploading
- Django - Apache Setup
- Django - Cookies Handling
- Django - Sessions
- Django - Caching
- Django - Comments
- Django - RSS
- Django - AJAX
- Django Useful Resources
- Django - Quick Guide
- Django - Useful Resources
- Django - Discussion
Django Admin - Create User
The Django framework readily provides a robust user management system. The users, groups, and permissions are managed both through the admin interface as well as programmatically.
When you create a new project with the startproject command, the admin and auth apps are added in the INSTALLED_APPS by default. All the user objects are stored in the "django.contrib.auth.models.User" model.
Assuming that the project has been created with the following command −
django-admin startproject myproject
The admin and auth apps are found in the list of INSTALLED_APPS in the settings module −
INSTALLED_APPS = [ 'django.contrib.admin', 'django.contrib.auth', . . ., ]
You need at least one user to be able to use the admin panel. The User objects are of the following types −
Superuser − A user object that can log into the admin site and possesses permissions to add/change/delete other users as well as perform CRUD operations on all the models in the project, through the admin interface itself.
Staff − The User object has a is_staff property. When this property is set to True, the user can login to the Django admin site. The superuser is a staff user by default.
Active − All users are marked as "active" by default. A normal active user (without staff privilege) is not authorized to use the Admin site.
Creating a Superuser
A superuser can be created in two ways.
With createsuperuser parameter to manage.py script from the command line
Calling create_superuser() function programmatically.
The command line use is straightforward. Execute following command from inside the project’s parent folder −
python manage.py createsuperuser
Enter the username and password when prompted −
Username: admin Email address: admin@example.com Password: ******** Password (again): ******** The password is too similar to the username. This password is too short. It must contain at least 8 characters. This password is too common. Bypass password validation and create user anyway? [y/N]: y Superuser created successfully.
Start the Django server, and login with the superuser credentials, open the login page of admin site http://localhost:8000/admin/.
The admin homepage shows the two models Groups and Users.
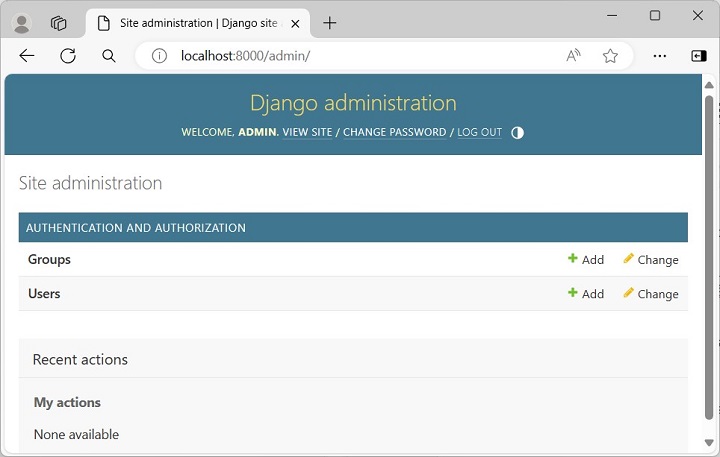
Creating a New User
To create a new user, click the "+" Add button. Enter the Username (manager) and the password that complies with the rules as mentioned.
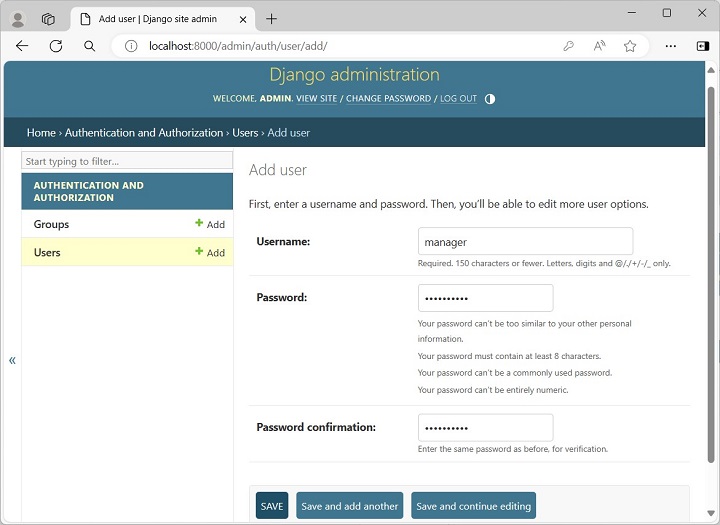
After the new user is successfully added, scroll down to enable the staff status if you want to grant the privilege of logging in. By default, each user is Active. You can also enter the other details such as the name and email address, etc.
Expand the Users model to see all the users.
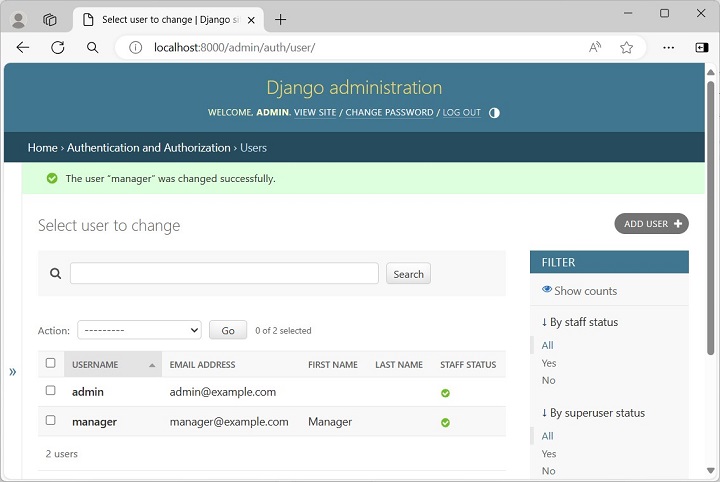
To create the user programmatically, use the Django shell.
Call the create_user() function as follows −
>>> from django.contrib.auth.models import User >>> usr=User.objects.create_user('testusr', 'test@example.com', 'pass123')
To accord the staff status, set the is_staff property to True.
>>> usr.is_staff=True >>> usr.save()
Refresh the users list in the admin site. You should see the newly added user.
A group categorizes users so you can apply permissions, or some other label, to those users. A user can belong to any number of groups.
In Django, a group is a list of permissions to be assigned to one or more users. When you create or modify a user, simply choose the desired group, so that all the permissions listed in it will be accorded to the user. In addition, you can always add other permissions to a user in addition to those obtained from the user.
Go to the homepage of the admin site and click the Add button in Groups model row to add a new group called admins. Select the permissions to be accorded to the users in this group from the list of available permissions.
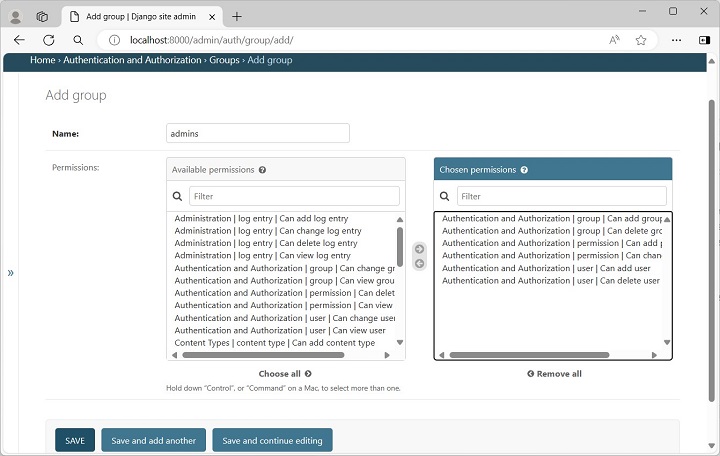
To enable all the permissions included in this group to a user, modify his properties and scroll down to see the available groups, make the selection and save.
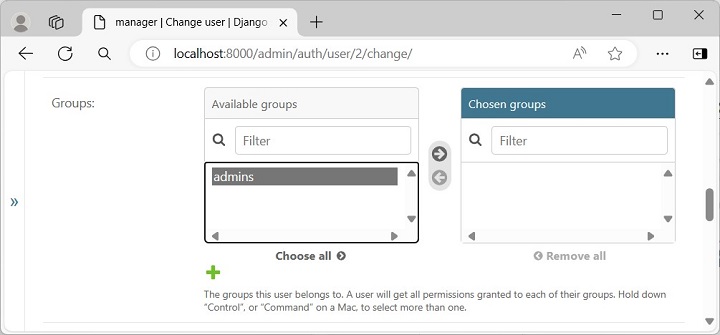
Django’s admin site can be very effectively used to create and manage users as well as manage groups and permissions.
To Continue Learning Please Login