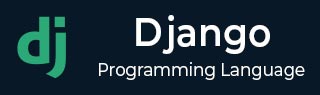
- Django Basic Concepts
- Django - Home
- Django - Basics
- Django - Overview
- Django - Environment
- Django - Creating a Project
- Django - Apps Life Cycle
- Django - Creating Views
- Django - URL Mapping
- Django - Templates System
- Django - MVT
- Django Admin
- Django Admin - Interface
- Django Admin - Create User
- Django Admin - Include Models
- Django Admin - Set Fields to Display
- Django Admin - Update Objects
- Django Models
- Django - Models
- Django - Insert Data
- Django - Update Data
- Django - Delete Data
- Django - Update Model
- Django Advanced
- Django - Page Redirection
- Django - Sending E-mails
- Django - Generic Views
- Django - Form Processing
- Django - File Uploading
- Django - Apache Setup
- Django - Cookies Handling
- Django - Sessions
- Django - Caching
- Django - Comments
- Django - RSS
- Django - AJAX
- Django Useful Resources
- Django - Quick Guide
- Django - Useful Resources
- Django - Discussion
Django – Delete Data
Django ORM is an important feature of Django framework. It is an abstract layer for the interaction between a model class and its mapped database table. An instance of the model class corresponds to a single row in the table. We can perform all the CRUD operations on the object; it will be automatically reflected in its mapped row.
In this chapter, we shall find out how we can delete an object and thereby an already existing row in a relational database.
We shall use the Dreamreal model as given below for the exercise −
class Dreamreal(models.Model): website = models.CharField(max_length=50) mail = models.CharField(max_length=50) name = models.CharField(max_length=50) phonenumber = models.IntegerField() def __str__(self): return "Website: {} Email: {} Name: {} Ph.: {}".format(self.website, self.mail, self.name, self.phonenumber)
It is assumed that you have already performed the migrations and added a few objects in the model.
Delete Object From Shell
Django has a useful feature with which you can invoke a Python shell inside the Django project’s environment.
Use the shell command with the manage.py script −
python manage.py shell
In front of the Python prompt, import the Dreamreal model −
>>> from myapp.models import Dreamreal
You can delete one or more objects from the model with the delete() method. This method can be applied on the QuerySet that consists of more than one objects, or on a single instance.
To delete a single object, do it from the model with get() method of the objects() manager −
row = Dreamreal.objects.get(pk = 1)
You can now invoke the delete() method −
row.delete()
The following statement returns a QuerySet of objects with name starting with "a" −
rows = Dreamreal.objects.filter(name__startswith = 'a')
You can perform bulk delete operation by calling delete() method on the queryset −
rows.delete()
Django returns the number of objects deleted and a dictionary with the number of deletions per object type.
In case of related tables, Django’s ForeignKey emulates the SQL constraint ON DELETE CASCADE by default. In other words, any objects with foreign keys pointing at the objects to be deleted will be deleted along with them.
Perform Delete Operation by Calling a View Function
Let us now perform the delete operation by calling a view function. Define update() function in views.py file.
This function receives the primary key as the argument from its mapped URL.
from django.shortcuts import render from django.http import HttpResponse from myapp.models import Dreamreal def delete(request, pk): obj = Dreamreal.objects.get(pk=pk) obj.delete() return HttpResponse("Deleted successfully")
We also need to register this view in the urls.py file by adding a new path.
urlpatterns + = [path("delete/<int:pk>", views.delete, name='delete')]
After making these changes, run Django server and visit http://localhost:8000/myapp/delete/2. The server responds with the deleted successfully message.
If you would like to access an object with a given name instead of primary key, make changes in the delete() view as follows −
def delete(request, name): obj = Dreamreal.objects.get(name=name) obj.delete() return HttpResponse("Deleted successfully")
Also change the URL mapping of the delete() view in URLCONFIG of myapp −
urlpatterns + = [path("delete/<name>", views.delete, name='delete')]
The delete operation must be carried out with caution, as you can accidentally delete data that you don’t intend to. Hence, we can ask a confirmation from the user. For this purpose, the delete() view renders a form with submit and cancel buttons.
def delete(request, pk): obj = Dreamreal.objects.get(pk=pk) if request.method == "POST": obj.delete() obj.save() return HttpResponse("<h2>Record updated Successfully</h2>") obj = Dreamreal.objects.get(pk=pk) context = {"pk":pk} return render(request, "myform.html", context)
The myform.html in templates folder has two buttons. The submit button posts back to delete() view, and calls the delete() method on the object −
<html> <body> <form action="../delete/{{ pk }}" method="post"> {% csrf_token %} <h2>The record with primary {{ pk }} will be deleted. Are you sure?</h2> <input type="submit" value="delete"> <a href = "../cancel"><input type="button" value="Cancel" /></a> </form> </body> </html>
Add the cancel() view as follows −
def cancel(request): return HttpResponse("<h2>Delete operation Cancelled by the user</h2>")
Visit the http://localhost:8000/myapp/delete/2 URL.
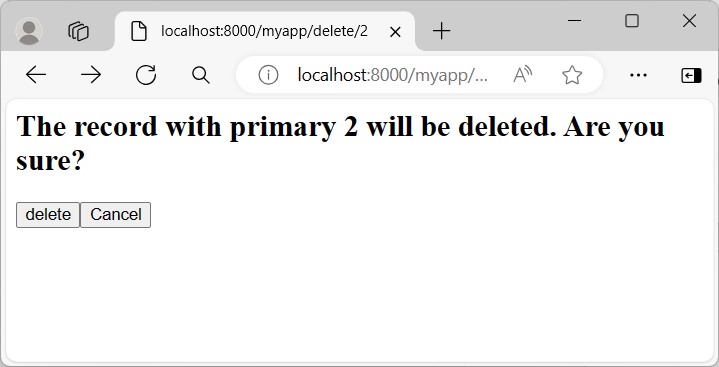
If the user presses the Cancel button, the cancel() view displays this message −
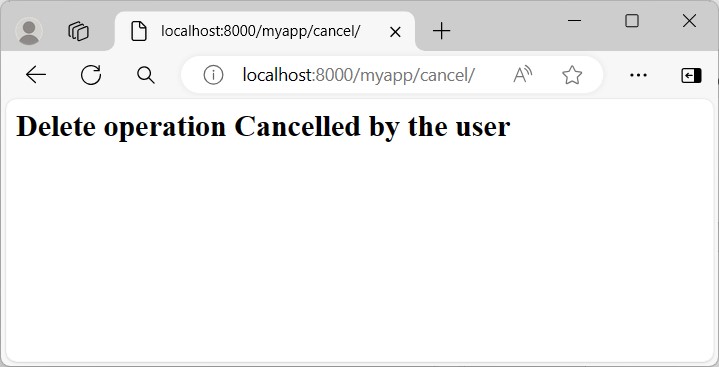
Otherwise, the record will be deleted from the table.
The DeleteView Class
Django defines a collection of generic view classes. The DeleteView class is specially designed for performing the DELETE query operation.
We define a subclass of DeleteView class, and set its template_name property to myform.html that we have already created.
Add the following code in views.py file −
from django.views.generic.edit import DeleteView class DRDeleteView(DeleteView): model = Dreamreal template_name = "myform.html" success_url = "../success/"
The myform.html file remains the same −
<html> <body> <form method="post"> {% csrf_token %} <h2>The record will be deleted. Are you sure?</h2> <input type="submit" value="delete"> <a href = "../cancel"> <input type="button" value="Cancel" /></a> </form> </body> </html>
Add the mapping to the DeleteView generic class in urls.py file −
from .views import DRDeleteView urlpatterns += [path("deleteview/<int:pk>", DRDeleteView.as_view(), name='deleteview')]
Check that the desired record is deleted by visiting http://localhost:8000/myapp/deleteview/2 URL.
In this chapter, we explained how you can delete data from Django model using different methods.
To Continue Learning Please Login