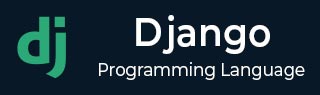
- Django Basic Concepts
- Django - Home
- Django - Basics
- Django - Overview
- Django - Environment
- Django - Creating a Project
- Django - Apps Life Cycle
- Django - Creating Views
- Django - URL Mapping
- Django - Templates System
- Django - MVT
- Django Admin
- Django Admin - Interface
- Django Admin - Create User
- Django Admin - Include Models
- Django Admin - Set Fields to Display
- Django Admin - Update Objects
- Django Models
- Django - Models
- Django - Insert Data
- Django - Update Data
- Django - Delete Data
- Django - Update Model
- Django Advanced
- Django - Page Redirection
- Django - Sending E-mails
- Django - Generic Views
- Django - Form Processing
- Django - File Uploading
- Django - Apache Setup
- Django - Cookies Handling
- Django - Sessions
- Django - Caching
- Django - Comments
- Django - RSS
- Django - AJAX
- Django Useful Resources
- Django - Quick Guide
- Django - Useful Resources
- Django - Discussion
Django – Insert Data
Django has its own Object Relation Model (ORM) that maps a Python class with a table in a relational database. The ORM mechanism helps in performing CRUD operations in object-oriented manner. In this chapter, we shall learn about different methods of inserting a new data.
By default, Django uses a SQLite database. A model in Django is a class inherited from the "django.db.models" class.
Let us use the following Dreamreal model in "models.py" file for learning how to insert data in the mapped table −
from django.db import models class Dreamreal(models.Model): website = models.CharField(max_length=50) mail = models.CharField(max_length=50) name = models.CharField(max_length=50) phonenumber = models.IntegerField() class Meta: db_table = "dreamreal"
After declaring a model, we need to perform the migrations −
python manage.py makemigrations python manage.py migrate
Insert Object From Shell
Django has a useful feature with which you can invoke a Python shell inside the Django project’s environment. Use the shell command with the manage.py script −
python manage.py shell
In front of the Python prompt, import the Dreamreal model −
>>> from myapp.models import Dreamreal
We can construct an object of this class and call its save() method so that the corresponding row is added in the table
>>> obj = Dreamreal(website="www.polo.com", mail="sorex@polo.com", name="sorex", phonenumber="002376970") >>> obj.save()
We can confirm this by opening the database in any SQLite viewer.
You can also use the create() method of the objects attribute of the model class to insert the record.
>>> obj = Dreamreal.objects.create(website="www.polo.com", mail="sorex@polo.com", name="sorex", phonenumber="002376970")
Perform Insert Operation by Calling a View Function
Let us now perform the insert operation by calling a View function. Define addnew() function in views.py file as follows −
from myapp.models import Dreamreal def addnew(request): obj = Dreamreal(website="www.polo.com", mail="sorex@polo.com", name="sorex", phonenumber="002376970") obj.save() return HttpResponse("<h2>Record Added Successfully")
We need to register this View with the URLpatterns list.
from django.urls import path from . import views urlpatterns = [ path("", views.index, name="index"), path("addnew/", views.addnew, name='addnew') ]
Instead of using the hard-coded values as in the above example, we would like the data to be accepted from the user. For this purpose, create an HTML form in myform.html file.
<html> <body> <form action="../addnew/" method="post"> {% csrf_token %} <p><label for="website">WebSite: </label> <input id="website" type="text" name="website"></p> <p><label for="mail">Email: </label> <input id="mail" type="text" name="mail"></p> <p><label for="name">Name: </label> <input id="name" type="text" name="name"></p> <p><label for="phonenumber">Phone Number: </label> <input id="phonenumber" type="text" name="phonenumber"></p> <input type="submit" value="OK"> </form> </body> </html>
The HTML template file must be stored in a directory defined in the TEMPLATES setting −
TEMPLATES = [ { 'BACKEND': 'django.template.backends.django.DjangoTemplates', 'DIRS': [BASE_DIR/'templates'], .. . ., ]
Let us use the render() function to display the form template −
from django.shortcuts import render from myapp.models import Dreamreal def addnew(request): if request.method == "POST": ws = request.POST['website'] mail = request.POST['mail'] nm = request.POST['name'] ph = request.POST['phonenumber'] obj = Dreamreal(website=ws, mail=mail, name=nm, phonenumber=ph) obj.save() return HttpResponse("<h2>Record Added Successfully</h2>") return render(request, "myform.html")
Note that the form’s action attribute is also set to the same URL mapping the addnew() function. Hence, we need to check the request.method. If it’s GET method, the blank form is rendered. If it’s POST method, the form data is parsed and used for inserting a new record.
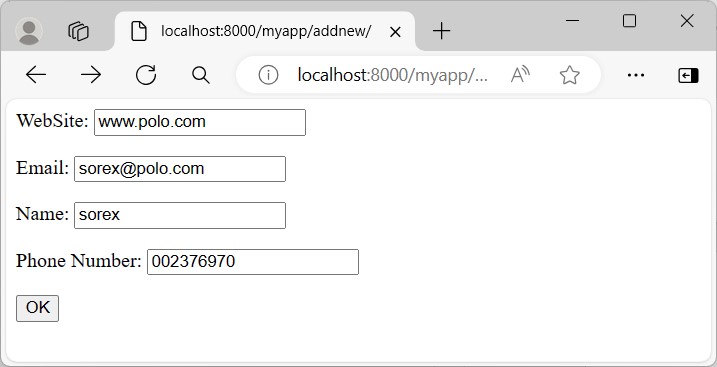
The Model Form
Django has a ModelForm class that automatically renders a HTML form with its structure matching with the attributes of a model class.
We define a DreamRealForm class in forms.py file under the app folder that uses the Dreamreal model as the basis.
from django import forms from .models import Dreamreal class DreamrealForm(forms.ModelForm): class Meta: model = Dreamreal fields = "__all__"
An object of the model form is rendered on the HTML form. In case the request method is POST, the save() method of the ModelForm class automatically validates the form and saves a new record.
from .forms import DreamrealForm def addnew(request): if request.method == 'POST': form = DreamrealForm(request.POST) if form.is_valid(): form.save() return HttpResponse("<h2>Book added successfully</h2>") context={'form' : DreamrealForm} return render(request, "myform.html", context)
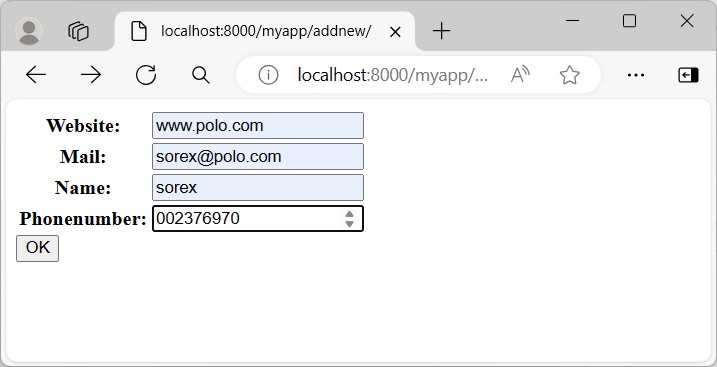
The CreateView Class
Django defines a collection of generic view classes. The CreateView class is specially designed for performing the INSERT query operation.
We define a subclass of CreateView class and set its template_name property to myform.html that we have already created.
Add the following code in views.py file −
from django.views.generic import CreateView class DRCreateView(CreateView): model = Dreamreal fields = "__all__" template_name = 'myform.html' success_url = '../success/'
On successful insertion, the browser is redirected to the success() view function.
def success(request): return HttpResponse("<h2>Book added successfully</h2>")
Both the above views must be included in the URL pattern list of urls.py file. The generic view classes are registered with their as_view() method.
from django.urls import path from . import views from .views import DRCreateView urlpatterns = [ path("", views.index, name="index"), path("addnew/", views.addnew, name='addnew'), path("add/", DRCreateView.as_view(), name='add'), path("success/", views.success, name='success'), ]
In this chapter, we learned the different ways to insert data in a Django model.
To Continue Learning Please Login