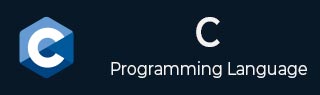
- C Programming Tutorial
- C - Home
- C - Overview
- C - Features
- C - History
- C - Environment Setup
- C - Program Structure
- C - Hello World
- C - Compilation Process
- C - Comments
- C - Tokens
- C - Keywords
- C - Identifiers
- C - User Input
- C - Basic Syntax
- C - Data Types
- C - Variables
- C - Integer Promotions
- C - Type Conversion
- C - Booleans
- C - Constants
- C - Literals
- C - Escape sequences
- C - Format Specifiers
- C - Storage Classes
- C - Operators
- C - Arithmetic Operators
- C - Relational Operators
- C - Logical Operators
- C - Bitwise Operators
- C - Assignment Operators
- C - Unary Operators
- C - Increment and Decrement Operators
- C - Ternary Operator
- C - sizeof Operator
- C - Operator Precedence
- C - Misc Operators
- C - Decision Making
- C - if statement
- C - if...else statement
- C - nested if statements
- C - switch statement
- C - nested switch statements
- C - Loops
- C - While loop
- C - For loop
- C - Do...while loop
- C - Nested loop
- C - Infinite loop
- C - Break Statement
- C - Continue Statement
- C - goto Statement
- C - Functions
- C - Main Functions
- C - Function call by Value
- C - Function call by reference
- C - Nested Functions
- C - Variadic Functions
- C - User-Defined Functions
- C - Callback Function
- C - Return Statement
- C - Recursion
- C - Scope Rules
- C - Static Variables
- C - Global Variables
- C - Arrays
- C - Properties of Array
- C - Multi-Dimensional Arrays
- C - Passing Arrays to Function
- C - Return Array from Function
- C - Variable Length Arrays
- C - Pointers
- C - Pointers and Arrays
- C - Applications of Pointers
- C - Pointer Arithmetics
- C - Array of Pointers
- C - Pointer to Pointer
- C - Passing Pointers to Functions
- C - Return Pointer from Functions
- C - Function Pointers
- C - Pointer to an Array
- C - Pointers to Structures
- C - Chain of Pointers
- C - Pointer vs Array
- C - Character Pointers and Functions
- C - NULL Pointer
- C - void Pointer
- C - Dangling Pointers
- C - Dereference Pointer
- C - Near, Far and Huge Pointers
- C - Initialization of Pointer Arrays
- C - Pointers vs. Multi-dimensional Arrays
- C - Strings
- C - Array of Strings
- C - Special Characters
- C - Structures
- C - Structures and Functions
- C - Arrays of Structures
- C - Self-Referential Structures
- C - Nested Structures
- C - Unions
- C - Bit Fields
- C - Typedef
- C - Input & Output
- C - File I/O
- C - Preprocessors
- C - Header Files
- C - Type Casting
- C - Error Handling
- C - Variable Arguments
- C - Memory Management
- C - Command Line Arguments
- C Programming Resources
- C - Questions & Answers
- C - Quick Guide
- C - Useful Resources
- C - Discussion
Nested Functions in C
The term nesting, in programming context refers to enclosing a particular programming element inside another similar element. Just like nested loops, nested structures, etc., a nested function is a term used to describe the use of one or more functions inside another function.
What is Lexical Scoping?
In C language, defining a function inside another one is not possible. In short, nested functions are not supported in C. A function may only be declared (not defined) within another function.
When a function is declared inside another function, it is called lexical scoping. Lexical scoping is not valid in C because the compiler cannot reach the correct memory location of inner function.
Nested Functions Have Limited Use
Nested function definitions cannot access local variables of surrounding blocks. They can access only global variables. In C, there are two nested scopes: local and global. So, nested functions have limited use.
Example: Nested Function
If you want to create a nested function like the one shown below, then it will generate an error −
#include <stdio.h> int main(void){ printf("Main Function"); int my_fun(){ printf("my_fun function"); // Nested Function int nested(){ printf("This is a nested function."); } } nested(); }
Output
On running this code, you will get an error −
main.c:(.text+0x3d): undefined reference to `nested' collect2: error: ld returned 1 exit status
Trampolines for Nested Functions
Nested functions are supported as an extension in "GNU C". GCC implements taking the address of a nested function using a technique called trampolines.
A trampoline is a piece of code created at runtime when the address of a nested function is taken. It requires the function to be prefixed with the keyword auto in the declaration.
Example 1
Take a look at the following example −
#include <stdio.h> int main(){ auto int nested(); nested(); printf("In Main Function now\n"); int nested(){ printf("In the nested function now\n"); } printf("End of the program"); }
Output
When you run this code, it will produce the following output −
In the nested function now In Main Function now End of the program
Example 2
In thi program, a function square() is nested inside another function myfunction(). The nested function is declared with the auto keyword.
#include <stdio.h> #include <math.h> double myfunction (double a, double b); int main(){ double x = 4, y = 5; printf("Addition of squares of %f and %f = %f", x, y, myfunction(x, y)); return 0; } double myfunction (double a, double b){ auto double square (double c) { return pow(c,2); } return square (a) + square (b); }
Output
Run the code and check its output −
Addition of squares of 4.000000 and 5.000000 = 41.000000
Nested Functions: Points to Note
One needs to be aware of the following points while using nested functions −
- A nested function can access all the identifiers of the containing function that precede its definition.
- A nested function must not be called before the containing function exits.
- A nested function cannot use a goto statement to jump to a label in the containing function.
- Nested function definitions are permitted within functions in any block, mixed with the other declarations and statements in the block.
- If you try to call a nested function through its address after the containing function exits, it throws an error.
- A nested function always has no linkage. Declaring one with "extern" or "static" always produces errors.
To Continue Learning Please Login