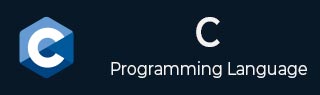
- C Programming Tutorial
- C - Home
- C - Overview
- C - Features
- C - History
- C - Environment Setup
- C - Program Structure
- C - Hello World
- C - Compilation Process
- C - Comments
- C - Tokens
- C - Keywords
- C - Identifiers
- C - User Input
- C - Basic Syntax
- C - Data Types
- C - Variables
- C - Integer Promotions
- C - Type Conversion
- C - Booleans
- C - Constants
- C - Literals
- C - Escape sequences
- C - Format Specifiers
- C - Storage Classes
- C - Operators
- C - Arithmetic Operators
- C - Relational Operators
- C - Logical Operators
- C - Bitwise Operators
- C - Assignment Operators
- C - Unary Operators
- C - Increment and Decrement Operators
- C - Ternary Operator
- C - sizeof Operator
- C - Operator Precedence
- C - Misc Operators
- C - Decision Making
- C - if statement
- C - if...else statement
- C - nested if statements
- C - switch statement
- C - nested switch statements
- C - Loops
- C - While loop
- C - For loop
- C - Do...while loop
- C - Nested loop
- C - Infinite loop
- C - Break Statement
- C - Continue Statement
- C - goto Statement
- C - Functions
- C - Main Functions
- C - Function call by Value
- C - Function call by reference
- C - Nested Functions
- C - Variadic Functions
- C - User-Defined Functions
- C - Callback Function
- C - Return Statement
- C - Recursion
- C - Scope Rules
- C - Static Variables
- C - Global Variables
- C - Arrays
- C - Properties of Array
- C - Multi-Dimensional Arrays
- C - Passing Arrays to Function
- C - Return Array from Function
- C - Variable Length Arrays
- C - Pointers
- C - Pointers and Arrays
- C - Applications of Pointers
- C - Pointer Arithmetics
- C - Array of Pointers
- C - Pointer to Pointer
- C - Passing Pointers to Functions
- C - Return Pointer from Functions
- C - Function Pointers
- C - Pointer to an Array
- C - Pointers to Structures
- C - Chain of Pointers
- C - Pointer vs Array
- C - Character Pointers and Functions
- C - NULL Pointer
- C - void Pointer
- C - Dangling Pointers
- C - Dereference Pointer
- C - Near, Far and Huge Pointers
- C - Initialization of Pointer Arrays
- C - Pointers vs. Multi-dimensional Arrays
- C - Strings
- C - Array of Strings
- C - Special Characters
- C - Structures
- C - Structures and Functions
- C - Arrays of Structures
- C - Self-Referential Structures
- C - Nested Structures
- C - Unions
- C - Bit Fields
- C - Typedef
- C - Input & Output
- C - File I/O
- C - Preprocessors
- C - Header Files
- C - Type Casting
- C - Error Handling
- C - Variable Arguments
- C - Memory Management
- C - Command Line Arguments
- C Programming Resources
- C - Questions & Answers
- C - Quick Guide
- C - Useful Resources
- C - Discussion
Variadic Functions in C
Variadic functions are one of the powerful but very rarely used features in C language. Variadic functions add a lot of flexibility in programming the application structure.
Variadic Function in C
A function that can take a variable number of arguments is called a variadic function. One fixed argument is required to define a variadic function.
The most frequently used library functions in C, i.e., printf() and scanf() are in fact the best-known examples of variadic functions, as we can put a variable number of arguments after the format specifier string.
printf("format specifier",arg1, arg2, arg3, . . .);
Syntax of Variadic Functions
In C, a variadic function is defined to have at least one fixed argument, and then followed by an ellipsis symbol (...) that enables the compiler to parse the variable number of arguments.
return_type function_name(type arg1, ...);
You need to include the stdarg.h header file at the top of the code to handle the variable arguments. This header file provides the following macros to work with the arguments received by the ellipsis symbol.
Methods | Description |
---|---|
va_start(va_list ap, arg) | Arguments after the last fixed argument are stored in the va_list. |
va_arg(va_list ap, type) | Each time, the next argument in the variable list va_list and coverts it to the given type, till it reaches the end of list. |
va_copy(va_list dest, va_list src) | This creates a copy of the arguments in va_list |
va_end(va_list ap) | This ends the traversal of the variadic function arguments. As the end of va_list is reached, the list object is cleaned up. |
Examples of Variadic Functions in C
Example: Sum of Numbers Using a Variadic Function
The following code uses the concept of a variadic function to return the sum of a variable number of numeric arguments passed to such a function. The first argument to the addition() function is the count of the remaining arguments.
Inside the addition() function, we initialize the va_list variable as follows −
va_list args; va_start (args, n);
Since there are "n" number of arguments that follow, fetch the next argument with "va_arg()" macro for "n" number of times and perform cumulative addition −
for(i = 0; i < n; i++){ sum += va_arg (args, int); }
Finally, clean up the va_list variable −
va_end (args);
The function ends by returning the sum of all the arguments.
The complete code is given below −
#include <stdio.h> #include <stdarg.h> // Variadic function to add numbers int addition(int n, ...){ va_list args; int i, sum = 0; va_start (args, n); for (i = 0; i < n; i++){ sum += va_arg (args, int); } va_end (args); return sum; } int main(){ printf("Sum = %d ", addition(5, 1, 2, 3, 4, 5)); return 0; }
Output
Run the code and check its output −
Sum = 15
You can try providing a different set of numbers as the variadic arguments to the addition() function.
Example: Finding the Largest Number Using a Variadic Function
We can extend the concept of variadic function to find the largest number in a given list of variable number of values.
#include <stdio.h> #include <stdarg.h> // Variadic function to add numbers int largest(int n, ...){ va_list args; int i, max = 0; va_start (args, n); for(i = 0; i < n; i++){ int x = va_arg (args, int); if (x >= max) max = x; } va_end (args); return max; } int main(){ printf("Largest number in the list = %d ", largest(5, 12, 34, 21, 45, 32)); return 0; }
Output
When you run this code, it will produce the following output −
Largest number in the list = 45
Example: Concatenation of Multiple Strings Using Variadic Function
In the following code, we pass multiple strings to a variadic function that returns a concatenated string.
#include <stdio.h> #include <string.h> #include <stdarg.h> char * concat(int n, ...){ va_list args; int i; static char string[100], *word; va_start (args, n); strcpy(string, ""); for (i = 0; i < n; i++){ word= va_arg (args, char *); strcat(string, word); strcat(string, " "); } va_end (args); return string; } int main(){ char * string1 = concat(2, "Hello", "World"); printf("%s\n", string1); char * string2 = concat(3, "How", "are", "you?"); printf("%s\n", string2); return 0; }
Output
Run the code and check its output −
Hello World How are you?
To Continue Learning Please Login