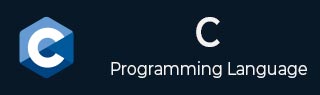
- C Programming Tutorial
- C - Home
- C - Overview
- C - Features
- C - History
- C - Environment Setup
- C - Program Structure
- C - Hello World
- C - Compilation Process
- C - Comments
- C - Tokens
- C - Keywords
- C - Identifiers
- C - User Input
- C - Basic Syntax
- C - Data Types
- C - Variables
- C - Integer Promotions
- C - Type Conversion
- C - Booleans
- C - Constants
- C - Literals
- C - Escape sequences
- C - Format Specifiers
- C - Storage Classes
- C - Operators
- C - Arithmetic Operators
- C - Relational Operators
- C - Logical Operators
- C - Bitwise Operators
- C - Assignment Operators
- C - Unary Operators
- C - Increment and Decrement Operators
- C - Ternary Operator
- C - sizeof Operator
- C - Operator Precedence
- C - Misc Operators
- C - Decision Making
- C - if statement
- C - if...else statement
- C - nested if statements
- C - switch statement
- C - nested switch statements
- C - Loops
- C - While loop
- C - For loop
- C - Do...while loop
- C - Nested loop
- C - Infinite loop
- C - Break Statement
- C - Continue Statement
- C - goto Statement
- C - Functions
- C - Main Functions
- C - Function call by Value
- C - Function call by reference
- C - Nested Functions
- C - Variadic Functions
- C - User-Defined Functions
- C - Callback Function
- C - Return Statement
- C - Recursion
- C - Scope Rules
- C - Static Variables
- C - Global Variables
- C - Arrays
- C - Properties of Array
- C - Multi-Dimensional Arrays
- C - Passing Arrays to Function
- C - Return Array from Function
- C - Variable Length Arrays
- C - Pointers
- C - Pointers and Arrays
- C - Applications of Pointers
- C - Pointer Arithmetics
- C - Array of Pointers
- C - Pointer to Pointer
- C - Passing Pointers to Functions
- C - Return Pointer from Functions
- C - Function Pointers
- C - Pointer to an Array
- C - Pointers to Structures
- C - Chain of Pointers
- C - Pointer vs Array
- C - Character Pointers and Functions
- C - NULL Pointer
- C - void Pointer
- C - Dangling Pointers
- C - Dereference Pointer
- C - Near, Far and Huge Pointers
- C - Initialization of Pointer Arrays
- C - Pointers vs. Multi-dimensional Arrays
- C - Strings
- C - Array of Strings
- C - Special Characters
- C - Structures
- C - Structures and Functions
- C - Arrays of Structures
- C - Self-Referential Structures
- C - Nested Structures
- C - Unions
- C - Bit Fields
- C - Typedef
- C - Input & Output
- C - File I/O
- C - Preprocessors
- C - Header Files
- C - Type Casting
- C - Error Handling
- C - Variable Arguments
- C - Memory Management
- C - Command Line Arguments
- C Programming Resources
- C - Questions & Answers
- C - Quick Guide
- C - Useful Resources
- C - Discussion
Relational Operators in C
Relational operators in C are defined to perform comparison of two values. The familiar angular brackets < and > are the relational operators in addition to a few more as listed in the table below.
These relational operators are used in Boolean expressions. All the relational operators evaluate to either True or False.
C doesn’t have a Boolean data type. Instead, "0" is interpreted as False and any non-zero value is treated as True.
Example 1
Here is a simple example of relational operator in C −
#include <stdio.h> int main(){ int op1 = 5; int op2 = 3; printf("op1: %d op2: %d op1 < op2: %d\n", op1, op2, op1 < op2); return 0; }
Output
Run the code and check its output −
op1: 5 op2: 3 op1 < op2: 0
Relational operators have an important role to play in decision-control and looping statements in C.
The following table lists all the relational operators in C −
Operator | Description | Example |
---|---|---|
== | Checks if the values of two operands are equal or not. If yes, then the condition becomes true. | (A == B) |
!= | Checks if the values of two operands are equal or not. If the values are not equal, then the condition becomes true. | (A != B) |
> | Checks if the value of left operand is greater than the value of right operand. If yes, then the condition becomes true. | (A > B) |
< | Checks if the value of left operand is less than the value of right operand. If yes, then the condition becomes true. | (A < B) |
>= | Checks if the value of left operand is greater than or equal to the value of right operand. If yes, then the condition becomes true. | (A >= B) |
<= | Checks if the value of left operand is less than or equal to the value of right operand. If yes, then the condition becomes true. | (A <= B) |
All the relational operators are binary operators. Since they perform comparison, they need two operands on either side.
We use the = symbol in C as the assignment operator. So, C uses the "==" (double equal) as the equality operator.
The angular brackets > and < are used as the "greater than" and "less than" operators. When combined with the "=" symbol, they form the ">=" operator for "greater than or equal" and "<=" operator for "less than or equal" comparison.
Finally, the "=" symbol prefixed with "!" (!=) is used as the inequality operator.
Example 2
The following example shows all the relational operators in use.
#include <stdio.h> int main(){ int a = 21; int b = 10; int c ; printf("a: %d b: %d\n", a,b); if(a == b){ printf("Line 1 - a is equal to b\n" ); } else { printf("Line 1 - a is not equal to b\n" ); } if (a < b){ printf("Line 2 - a is less than b\n" ); } else { printf("Line 2 - a is not less than b\n" ); } if (a > b){ printf("Line 3 - a is greater than b\n" ); } else { printf("Line 3 - a is not greater than b \n\n" ); } /* Lets change value of a and b */ a = 5; b = 20; printf("a: %d b: %d\n", a,b); if (a <= b){ printf("Line 4 - a is either less than or equal to b\n" ); } if (b >= a){ printf("Line 5 - b is either greater than or equal to b\n" ); } if(a != b){ printf("Line 6 - a is not equal to b\n" ); } else { printf("Line 6 - a is equal to b\n" ); } return 0; }
Output
When you run this code, it will produce the following output −
a: 21 b: 10 Line 1 - a is not equal to b Line 2 - a is not less than b Line 3 - a is greater than b a: 5 b: 20 Line 4 - a is either less than or equal to b Line 5 - b is either greater than or equal to b Line 6 - a is not equal to b
Example 3
The == operator needs to be used with care. Remember that "=" is the assignment operator in C. If used by mistake in place of the equality operator, you get an incorrect output as follows −
#include <stdio.h> int main(){ int a = 5; int b = 3; if (a = b){ printf("a is equal to b"); } else { printf("a is not equal to b"); } return 0; }
Output
The value of "b" is assigned to "a" which is non-zero, and hence the if expression returns true.
a is equal to b
Example 4
We can have "char" types too as the operand for all the relational operators, as the "char" type is a subset of "int" type. Take a look at this example −
#include <stdio.h> int main(){ char a = 'B'; char b = 'd'; printf("a: %c b: %c\n", a,b); if(a == b){ printf("Line 1 - a is equal to b \n"); } else { printf("Line 1 - a is not equal to b \n"); } if (a < b){ printf("Line 2 - a is less than b \n"); } else { printf("Line 2 - a is not less than b \n"); } if (a > b) { printf("Line 3 - a is greater than b \n"); } else { printf("Line 3 - a is not greater than b \n"); } if(a != b) { printf("Line 4 - a is not equal to b \n"); } else { printf("Line 4 - a is equal to b \n"); } return 0; }
Output
Run the code and check its output −
a: B b: d Line 1 - a is not equal to b Line 2 - a is less than b Line 3 - a is not greater than b Line 4 - a is not equal to b
Relational operators cannot be used for comparing secondary types such as arrays or derived types such as struct or union types.
To Continue Learning Please Login