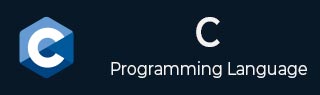
- C Programming Tutorial
- C - Home
- C - Overview
- C - Features
- C - History
- C - Environment Setup
- C - Program Structure
- C - Hello World
- C - Compilation Process
- C - Comments
- C - Tokens
- C - Keywords
- C - Identifiers
- C - User Input
- C - Basic Syntax
- C - Data Types
- C - Variables
- C - Integer Promotions
- C - Type Conversion
- C - Booleans
- C - Constants
- C - Literals
- C - Escape sequences
- C - Format Specifiers
- C - Storage Classes
- C - Operators
- C - Arithmetic Operators
- C - Relational Operators
- C - Logical Operators
- C - Bitwise Operators
- C - Assignment Operators
- C - Unary Operators
- C - Increment and Decrement Operators
- C - Ternary Operator
- C - sizeof Operator
- C - Operator Precedence
- C - Misc Operators
- C - Decision Making
- C - if statement
- C - if...else statement
- C - nested if statements
- C - switch statement
- C - nested switch statements
- C - Loops
- C - While loop
- C - For loop
- C - Do...while loop
- C - Nested loop
- C - Infinite loop
- C - Break Statement
- C - Continue Statement
- C - goto Statement
- C - Functions
- C - Main Functions
- C - Function call by Value
- C - Function call by reference
- C - Nested Functions
- C - Variadic Functions
- C - User-Defined Functions
- C - Callback Function
- C - Return Statement
- C - Recursion
- C - Scope Rules
- C - Static Variables
- C - Global Variables
- C - Arrays
- C - Properties of Array
- C - Multi-Dimensional Arrays
- C - Passing Arrays to Function
- C - Return Array from Function
- C - Variable Length Arrays
- C - Pointers
- C - Pointers and Arrays
- C - Applications of Pointers
- C - Pointer Arithmetics
- C - Array of Pointers
- C - Pointer to Pointer
- C - Passing Pointers to Functions
- C - Return Pointer from Functions
- C - Function Pointers
- C - Pointer to an Array
- C - Pointers to Structures
- C - Chain of Pointers
- C - Pointer vs Array
- C - Character Pointers and Functions
- C - NULL Pointer
- C - void Pointer
- C - Dangling Pointers
- C - Dereference Pointer
- C - Near, Far and Huge Pointers
- C - Initialization of Pointer Arrays
- C - Pointers vs. Multi-dimensional Arrays
- C - Strings
- C - Array of Strings
- C - Special Characters
- C - Structures
- C - Structures and Functions
- C - Arrays of Structures
- C - Self-Referential Structures
- C - Nested Structures
- C - Unions
- C - Bit Fields
- C - Typedef
- C - Input & Output
- C - File I/O
- C - Preprocessors
- C - Header Files
- C - Type Casting
- C - Error Handling
- C - Variable Arguments
- C - Memory Management
- C - Command Line Arguments
- C Programming Resources
- C - Questions & Answers
- C - Quick Guide
- C - Useful Resources
- C - Discussion
C - The sizeof Operator
The sizeof operator is a compile−time unary operator. It is used to compute the size of its operand, which may be a data type or a variable. It returns the size in number of bytes.
It can be applied to any data type, float type, or pointer type variables.
sizeof(type or var);
When sizeof() is used with a data type, it simply returns the amount of memory allocated to that data type. The outputs can be different on different machines, for example, a 32-bit system can show a different output as compared to a 64-bit system.
Example 1: Using the sizeof Operator in C
Take a look at the following example. It highlights how you can use the sizeof operator in a C program −
#include <stdio.h> int main(){ int a = 16; printf("Size of variable a: %d \n",sizeof(a)); printf("Size of int data type: %d \n",sizeof(int)); printf("Size of char data type: %d \n",sizeof(char)); printf("Size of float data type: %d \n",sizeof(float)); printf("Size of double data type: %d \n",sizeof(double)); return 0; }
Output
On running this code, you will get the following output −
Size of variable a: 4 Size of int data type: 4 Size of char data type: 1 Size of float data type: 4 Size of double data type: 8
Example 2: Using sizeof with Struct
In this example, we declare a struct type and find the size of the struct type variable.
#include <stdio.h> struct employee { char name[10]; int age; double percent; }; int main(){ struct employee e1 = {"Raghav", 25, 78.90}; printf("Size of employee variable: %d\n",sizeof(e1)); return 0; }
Output
Run the code and check its output −
Size of employee variable: 24
Example 3: Using sizeof with Array
In the following code, we have declared an array of 10 int values. Applying the sizeof operator on the array variable returns 40. This is because the size of an int is 4 bytes.
#include <stdio.h> int main(){ int arr[] = {1, 2, 3, 4, 5, 6, 7, 8, 9, 10}; printf("Size of arr: %d\n", sizeof(arr)); }
Output
Run the code and check its output −
Size of arr: 40
Example 4: Using sizeof to Find the Length of an Array
In C, we don’t have a function that returns the number of elements in a numeric array (we can get the number of characters in a string using the strlen() function). For this purpose, we can use the sizeof() operator.
We first find the size of the array and then divide it by the size of its data type.
#include <stdio.h> int main(){ int arr[] = {1, 2, 3, 4, 5, 6, 7, 8, 9, 10}; int y = sizeof(arr)/sizeof(int); printf("No of elements in arr: %d\n", y); }
Output
When you run this code, it will produce the following output −
No of elements in arr: 10
Example 5: Using sizeof in Dynamic Memory Allocation
The sizeof operator is used to compute the memory block to be dynamically allocated with the malloc() and calloc() functions.
The malloc() function is used with the following syntax −
type *ptr = (type *) malloc(sizeof(type)*number);
The following statement allocates a block of 10 integers and stores its address in the pointer −
int *ptr = (int *)malloc(sizeof(int)*10);
When the sizeof() is used with an expression, it returns the size of the expression. Here is an example.
#include <stdio.h> int main(){ char a = 'S'; double b = 4.65; printf("Size of variable a: %d\n",sizeof(a)); printf("Size of an expression: %d\n",sizeof(a+b)); int s = (int)(a+b); printf("Size of explicitly converted expression: %d\n",sizeof(s)); return 0; }
Output
Run the code and check its output −
Size of variable a: 1 Size of an expression: 8 Size of explicitly converted expression: 4
Example 6: The Size of a Pointer in C
The sizeof() operator returns the same value irrespective of the type. This includes the pointer of a built−in type, a derived type, or a double pointer.
#include <stdio.h> int main(){ printf("Size of int data type: %d \n", sizeof(int *)); printf("Size of char data type: %d \n", sizeof(char *)); printf("Size of float data type: %d \n", sizeof(float *)); printf("Size of double data type: %d \n", sizeof(double *)); printf("Size of double pointer type: %d \n", sizeof(int **)); }
Output
Run the code and check its output −
Size of int data type: 8 Size of char data type: 8 Size of float data type: 8 Size of double data type: 8 Size of double pointer type: 8
To Continue Learning Please Login