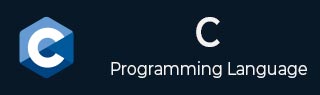
- C Programming Tutorial
- C - Home
- C - Overview
- C - Features
- C - History
- C - Environment Setup
- C - Program Structure
- C - Hello World
- C - Compilation Process
- C - Comments
- C - Tokens
- C - Keywords
- C - Identifiers
- C - User Input
- C - Basic Syntax
- C - Data Types
- C - Variables
- C - Integer Promotions
- C - Type Conversion
- C - Booleans
- C - Constants
- C - Literals
- C - Escape sequences
- C - Format Specifiers
- C - Storage Classes
- C - Operators
- C - Arithmetic Operators
- C - Relational Operators
- C - Logical Operators
- C - Bitwise Operators
- C - Assignment Operators
- C - Unary Operators
- C - Increment and Decrement Operators
- C - Ternary Operator
- C - sizeof Operator
- C - Operator Precedence
- C - Misc Operators
- C - Decision Making
- C - if statement
- C - if...else statement
- C - nested if statements
- C - switch statement
- C - nested switch statements
- C - Loops
- C - While loop
- C - For loop
- C - Do...while loop
- C - Nested loop
- C - Infinite loop
- C - Break Statement
- C - Continue Statement
- C - goto Statement
- C - Functions
- C - Main Functions
- C - Function call by Value
- C - Function call by reference
- C - Nested Functions
- C - Variadic Functions
- C - User-Defined Functions
- C - Callback Function
- C - Return Statement
- C - Recursion
- C - Scope Rules
- C - Static Variables
- C - Global Variables
- C - Arrays
- C - Properties of Array
- C - Multi-Dimensional Arrays
- C - Passing Arrays to Function
- C - Return Array from Function
- C - Variable Length Arrays
- C - Pointers
- C - Pointers and Arrays
- C - Applications of Pointers
- C - Pointer Arithmetics
- C - Array of Pointers
- C - Pointer to Pointer
- C - Passing Pointers to Functions
- C - Return Pointer from Functions
- C - Function Pointers
- C - Pointer to an Array
- C - Pointers to Structures
- C - Chain of Pointers
- C - Pointer vs Array
- C - Character Pointers and Functions
- C - NULL Pointer
- C - void Pointer
- C - Dangling Pointers
- C - Dereference Pointer
- C - Near, Far and Huge Pointers
- C - Initialization of Pointer Arrays
- C - Pointers vs. Multi-dimensional Arrays
- C - Strings
- C - Array of Strings
- C - Special Characters
- C - Structures
- C - Structures and Functions
- C - Arrays of Structures
- C - Self-Referential Structures
- C - Nested Structures
- C - Unions
- C - Bit Fields
- C - Typedef
- C - Input & Output
- C - File I/O
- C - Preprocessors
- C - Header Files
- C - Type Casting
- C - Error Handling
- C - Variable Arguments
- C - Memory Management
- C - Command Line Arguments
- C Programming Resources
- C - Questions & Answers
- C - Quick Guide
- C - Useful Resources
- C - Discussion
Strings in C
The C language does not have a "string" data type. A string in C is a one-dimensional array of char type, with the last character in the array being a "null character" represented by '\0'. Thus, a string in C can be defined as a null-terminated sequence of char type values.
Let us create a string "Hello". It comprises of five char values. In C, the literal representation of a char type uses single quote symbols − such as 'H'. These five alphabets put inside single quotes, followed by null character represented by '\0' are assigned to an array of char type. The size of the array is five characters plus the null character − six.
char greeting[6] = {'H', 'e', 'l', 'l', 'o', '\0'};
C lets you initialize an array without declaring the size, in which case the compiler automatically determines the array size.
char greeting[] = {'H', 'e', 'l', 'l', 'o', '\0'};
The array created in the memory can be schematically shown as follows −
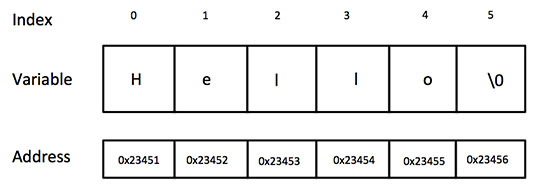
If the string is not terminated by "\0", it results in unpredictable behavior.
Note: The length of the string doesn’t include the null character. The library function strlen() returns the length of this string as 5.
Example: Creating a String in C
We can print the characters in this string array with a "for loop" as follows −
#include <stdio.h> #include <string.h> int main (){ char greeting[] = {'H', 'e', 'l', 'l', 'o', '\0'}; for (int i = 0; i < 5; i++) { printf("%c", greeting[i]); } return 0; }
Output
It will produce the following output −
Hello
The %s Format Specifier
C provides a format specifier "%s" which is used to print a string when you're using functions like printf or fprintf functions.
Example
The "%s" specifier tells the function to iterate through the array, until it encounters the null terminator (\0) and printing each character. This effectively prints the entire string represented by the character array without having to use a loop.
#include <stdio.h> int main (){ char greeting[] = {'H', 'e', 'l', 'l', 'o', '\0'}; printf("Greeting message: %s\n", greeting ); return 0; }
Output
It will produce the following output −
Greeting message: Hello
You can declare an oversized array and assign less number of characters, to which the compiler has no issues. However, if the size is less than the characters in the initialization, you may get garbage values in the output.
char greeting[3] = {'H', 'e', 'l', 'l', 'o', '\0'}; printf("%s", greeting);
Constructing a String using Double Quotes
Instead of constructing a char array of individual char values in single quotation marks, and using "\0" as the last element, C lets you construct a string by enclosing the characters within double quotation marks. This method of initializing a string is more convenient, as the compiler automatically adds "\0" as the last character.
char greeting[] = "Hello World";
String Input
Declaring a null−terminated string causes difficulty if you want to ask the user to input a string. You can accept one character at a time to store in each subscript of an array, with the help of a loop −
for(i = 0; i < 6; i++){ scanf("%c", &greeting[i]); }
Example
It is not possible to input "\0" (the null string) because it is a non-printable character. To overcome this, the "%s" format specifier is used in the scanf() statement −
#include <stdio.h> #include <string.h> int main (){ char greeting[10]; printf("Enter a string:\n"); scanf("%s", greeting); printf("You entered: \n"); printf("%s", greeting); return 0; }
Output
Run the code and check its output −
Enter a string: Hello You entered: Hello
Note: If the size of the array is less than the length of the input string, then it may result in situations such as garbage, data corruption, etc.
String Input with Whitespace
scanf("%s") reads characters until it encounters a whitespace (space, tab, newline, etc.) or EOF. So, if you try to input a string with multiple words (separated by whitespaces), then the C program would accept characters before the first whitespace as the input to the string.
Example
Take a look at the following example −
#include <stdio.h> #include <string.h> int main (){ char greeting[20]; printf("Enter a string:\n"); scanf("%s", greeting); printf("You entered: \n"); printf("%s", greeting); return 0; }
Output
Run the code and check its output −
Enter a string: Hello World! You entered: Hello
The gets() and fgets() Function
To accept a string input with whitespaces in between, we should use the gets() function. It is called an unformatted console input function, defined in the "stdio.h" header file.
Example 1
Take a look at the following example −
#include <stdio.h> #include <string.h> int main(){ char name[20]; printf("Enter a name:\n"); gets(name); printf("You entered: \n"); printf("%s", name); return 0; }
Output
Run the code and check its output −
Enter a name: Sachin Tendulkar You entered: Sachin Tendulkar
In newer versions of C, gets() has been deprecated. It is potentially a dangerous function because it doesn’t perform bound checks and may result in buffer overflow.
Instead, it is advised to use the fgets() function.
fgets(char arr[], size, stream);
fgets() can be used to accept input from any input stream, such as stdin (keyboard) or FILE (file stream).
Example 2
The following program uses fgets() and accepts multiword input from the user.
#include <stdio.h> #include <string.h> int main(){ char name[20]; printf("Enter a name:\n"); fgets(name, sizeof(name), stdin); printf("You entered: \n"); printf("%s", name); return 0; }
Output
Run the code and check its output −
Enter a name: Virat Kohli You entered: Virat Kohli
Example 3
You may also use scanf("%[^\n]s") as an alternative. It reads the characters until a newline character ("\n") is encountered.
#include <stdio.h> #include <string.h> int main (){ char name[20]; printf("Enter a name: \n"); scanf("%[^\n]s", name); printf("You entered \n"); printf("%s", name); return 0; }
Output
Run the code and check its output −
Enter a name: Zaheer Khan You entered Zaheer Khan
puts() and fputs()
We have been using printf() function with %s specifier to print a string. We can also use puts() function (deprecated in C11 and C17 versions) or fputs() functions as an alternative.
Example
Take a look at the following example −
#include <stdio.h> #include <string.h> int main (){ char name[20] = "Rakesh Sharma"; printf("With puts(): \n"); puts(name); printf("With fputs(): \n"); fputs(name, stdout); return 0; }
Output
Run the code and check its output −
With puts(): Harbhajan Singh With fputs(): Harbhajan Singh
To Continue Learning Please Login