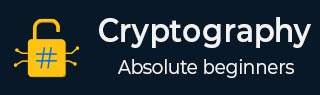
- Cryptography Tutorial
- Cryptography - Home
- Cryptography - Origin
- Cryptography - History
- Cryptography - Principles
- Cryptography - Applications
- Cryptography - Benefits & Drawbacks
- Cryptography - Modern Age
- Cryptography - Traditional Ciphers
- Cryptography - Need for Encryption
- Cryptography - Double Strength Encryption
- Cryptosystems
- Cryptosystems
- Cryptosystems - Components
- Attacks On Cryptosystem
- Cryptosystems - Rainbow table attack
- Cryptosystems - Dictionary attack
- Cryptosystems - Brute force attack
- Cryptosystems - Cryptanalysis Techniques
- Types of Cryptography
- Cryptosystems - Types
- Public Key Encryption
- Modern Symmetric Key Encryption
- Cryptography Hash functions
- Key Management
- Cryptosystems - Key Generation
- Cryptosystems - Key Storage
- Cryptosystems - Key Distribution
- Cryptosystems - Key Revocation
- Block Ciphers
- Cryptosystems - Stream Cipher
- Cryptography - Block Cipher
- Cryptography - Feistel Block Cipher
- Block Cipher Modes of Operation
- Block Cipher Modes of Operation
- Electronic Code Book (ECB) Mode
- Cipher Block Chaining (CBC) Mode
- Cipher Feedback (CFB) Mode
- Output Feedback (OFB) Mode
- Counter (CTR) Mode
- Classic Ciphers
- Cryptography - Reverse Cipher
- Cryptography - Caesar Cipher
- Cryptography - ROT13 Algorithm
- Cryptography - Transposition Cipher
- Cryptography - Encryption Transposition Cipher
- Cryptography - Decryption Transposition Cipher
- Cryptography - Multiplicative Cipher
- Cryptography - Affine Ciphers
- Cryptography - Simple Substitution Cipher
- Cryptography - Encryption of Simple Substitution Cipher
- Cryptography - Decryption of Simple Substitution Cipher
- Cryptography - Vigenere Cipher
- Cryptography - Implementing Vigenere Cipher
- Modern Ciphers
- Base64 Encoding & Decoding
- Cryptography - XOR Encryption
- Substitution techniques
- Cryptography - MonoAlphabetic Cipher
- Cryptography - Hacking Monoalphabetic Cipher
- Cryptography - Polyalphabetic Cipher
- Cryptography - Playfair Cipher
- Cryptography - Hill Cipher
- Polyalphabetic Ciphers
- Cryptography - One-Time Pad Cipher
- Implementation of One Time Pad Cipher
- Cryptography - Transposition Techniques
- Cryptography - Rail Fence Cipher
- Cryptography - Columnar Transposition
- Cryptography - Steganography
- Symmetric Algorithms
- Cryptography - Data Encryption
- Cryptography - Encryption Algorithms
- Cryptography - Data Encryption Standard
- Cryptography - Triple DES
- Cryptography - Double DES
- Advanced Encryption Standard
- Cryptography - AES Structure
- Cryptography - AES Transformation Function
- Cryptography - Substitute Bytes Transformation
- Cryptography - ShiftRows Transformation
- Cryptography - MixColumns Transformation
- Cryptography - AddRoundKey Transformation
- Cryptography - AES Key Expansion Algorithm
- Cryptography - Blowfish Algorithm
- Cryptography - SHA Algorithm
- Cryptography - RC4 Algorithm
- Cryptography - Camellia Encryption Algorithm
- Cryptography - ChaCha20 Encryption Algorithm
- Cryptography - CAST5 Encryption Algorithm
- Cryptography - SEED Encryption Algorithm
- Cryptography - SM4 Encryption Algorithm
- IDEA - International Data Encryption Algorithm
- Public Key (Asymmetric) Cryptography Algorithms
- Cryptography - RSA Algorithm
- Cryptography - RSA Encryption
- Cryptography - RSA Decryption
- Cryptography - Creating RSA Keys
- Cryptography - Hacking RSA Cipher
- Cryptography - ECDSA Algorithm
- Cryptography - DSA Algorithm
- Cryptography - Diffie-Hellman Algorithm
- Data Integrity in Cryptography
- Data Integrity in Cryptography
- Message Authentication
- Cryptography Digital signatures
- Public Key Infrastructure
- Cryptography Useful Resources
- Cryptography - Quick Guide
- Cryptography - Discussion
Cryptography - Caesar Cipher
So the next cryptographic algorithm is Caesar Cipher. In this chapter we will see what exactly Caesar Cipher is, how it works and also its implementations with different techniques. So let us deep dive into it.
What is a Caesar Cipher ?
The Caesar Cipher algorithm is the simple and easy approach of encryption technique. It is a simple type of substitution cipher in which the alphabets are moved by using a selected number of areas to create the encoded message. An A can be encoded as a C, M as an O, a Z as an B, and so on with the usage of a Caesar cipher with a shift of 2. This technique is named after Roman leader Julius Caesar. It is used in his private correspondence. It is one of the simplest and oldest methods to encrypt messages.
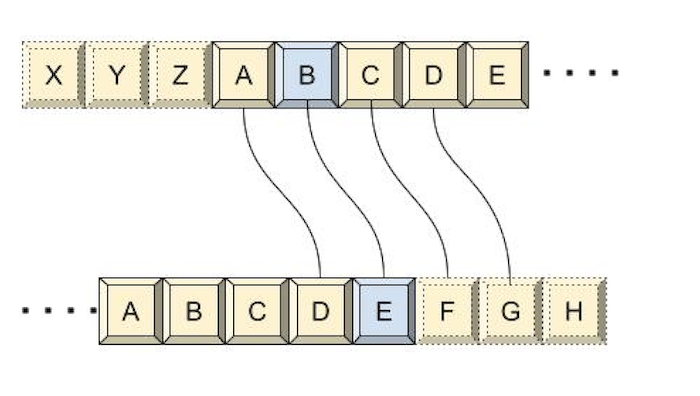
Algorithm
Here's the algorithm for the Caesar Cipher for encryption and decryption both −
Encryption Algorithm
For encryption algorithm the steps are as follows −
Choose a number to be your "shift" value. This number decides how much each letter will move in the alphabet.
Start with your message. Look at each letter in your message.
Move each letter forward in the alphabet by the chosen shift value. For example, if the shift value is 3, then "A" will become "D", "M" will become "P", and so on.
Save the new letter instead of the old one.
Continue this for every letter in the message.
Decryption Algorithm
For the decryption algorithm see the steps below −
Start with the encrypted message.
Know the shift value used for encryption.
- Look at each letter in the encrypted message.
Move each letter back in the alphabet by the shift value to decrypt it. For example, if the shift value is 3, then "D" will become "A", "P" will become "M", and so on.
Save the decrypted letter instead of the encrypted one.
Continue this for all the letters in the encrypted message.
Implementation Using Python
So, using various Python modules and methods, we can implement this algorithm in multiple ways. In the sections below, let us explore each of these methods individually −
Using the String Module
Using the List Comprehension
Using the String Module
In this approach we are going to use the string module of Python. This module is used to work with strings. This module has some constants, utility functions, and classes for string manipulation. As it is a built-in module so we will have to import it before using any of its constants and classes.
So the string module is used to access the lowercase alphabet and perform string manipulation operations necessary for creating the Caesar Cipher algorithm.
Encryption Example
Below is the implementation of Caesar Cipher using string module of Python −
import string def caesar_cipher(text, shift): letters = string.ascii_lowercase shifted_letters = letters[shift:] + letters[:shift] table = str.maketrans(letters, shifted_letters) return text.translate(table) # function execution message = "hello" shift_value = 3 encrypted_msg = caesar_cipher(message, shift_value) print("Encrypted message:", encrypted_msg)
Following is the output of the above example −
Input/Output
I/P -> Plain text : hello O/P -> Encrypted message: khoor
Decryption Example
To decrypt the above text message we can use the below code in Python −
def caesar_decipher(text, shift): # Decryption is just like encryption but with a negative shift return caesar_cipher(text, -shift) # Decryption encrypted_msg = khoor decrypted_msg = caesar_decipher(encrypted_msg, shift_value) print("Decrypted message:", decrypted_msg)
Following is the output of the above example −
Input/Output
I/P -> Cipher Text: khoor O/P -> Decrypted message: hello
Using the List Comprehension
Now we are going to use list comprehension to create a new string via iterating over each person within the input text. Inside the listing, there is a conditional expression that checks if each letter is uppercase, lowercase, or non-alphabetical. We will basically encrypt every alphabetical letter within the input text and leave away the non-alphabetical characters as it is.
Encryption Example
Here is the implementation of the Caesar Cipher algorithm using list comprehension in Python −
def caesar_cipher(text, shift): encrypted_text = '' for char in text: if 'A' <= char <= 'Z': encrypted_text += chr((ord(char) - 65 + shift) % 26 + 65) elif 'a' <= char <= 'z': encrypted_text += chr((ord(char) - 97 + shift) % 26 + 97) else: encrypted_text += char return encrypted_text # function execution message = "hello everyone" shift_value = 3 encrypted_msg = caesar_cipher(message, shift_value) print("Encrypted message:", encrypted_msg)
Following is the output of the above example −
Input/Output
I/P -> Plain text: hello everyone O/P -> Encrypted message: khoor hyhubrqh
Decryption Example
To create the decryption program for the Caesar Cipher encrypted message, we can reverse the encryption process. Here's the decryption code for the above Caesar Cipher encryption function using list comprehension −
def caesar_decipher(text, shift): decrypted_text = '' for char in text: if 'A' <= char <= 'Z': decrypted_text += chr((ord(char) - 65 - shift) % 26 + 65) elif 'a' <= char <= 'z': decrypted_text += chr((ord(char) - 97 - shift) % 26 + 97) else: decrypted_text += char return decrypted_text # Function execution encrypted_msg = "khoor hyhubrqh" shift_value = 3 # Decryption decrypted_msg = caesar_decipher(encrypted_msg, shift_value) print("Decrypted message:", decrypted_msg)
Following is the output of the above example −
Input/Output
I/P -> Cipher text: khoor hyhubrqh O/P -> Decrypted message: hello everyone
Implementation using C++
This approach takes a message and a shift value as an input. It then iterates over each character inside the message and shifts it over the given amount to create Caesar Cipher algorithm. It returns the encrypted message as a string. So below is the implementation of Caesar Cipher using C++ programming langugage −
Example
#include <iostream> #include <string> using namespace std; // Function to encrypt a message string encrypt(string message, int shift) { string encrypted = ""; for (char& c : message) { // Shift each character by the given spaces if (isalpha(c)) { if (isupper(c)) { encrypted += char(int(c + shift - 65) % 26 + 65); } else { encrypted += char(int(c + shift - 97) % 26 + 97); } } else { encrypted += c; // Keep non-alphabetic characters unchanged } } return encrypted; } int main() { string message = "Hello, Tutorialspoint!"; int shift = 3; string encrypted_msg = encrypt(message, shift); cout << "The Original Message: " << message << endl; cout << "The Encrypted Message: " << encrypted_msg << endl; return 0; }
Following is the output of the above example −
Input/Output
The Original Message: Hello, Tutorialspoint! The Encrypted Message: Khoor, Wxwruldovsrlqw!
Implementation using Java
The input for this method is a shift value and a plaintext message. Then, with the help of the Caesar Cipher method we will iteratively shifts each character in the message by the given amount. The encrypted message is returned as a string. So the implementation in Java for Caesar Cipher is as follows −
Example
public class CaesarCipherClass { // Method to encrypt a message public static String encryptFunc(String message, int shift) { StringBuilder encrypted = new StringBuilder(); for (char c : message.toCharArray()) { // Shift each character by the given amount if (Character.isLetter(c)) { char base = Character.isUpperCase(c) ? 'A' : 'a'; encrypted.append((char) ((c - base + shift) % 26 + base)); } else { encrypted.append(c); // Keep non-alphabetic characters unchanged } } return encrypted.toString(); } public static void main(String[] args) { String message = "Hello, Everyone there!"; int shift = 3; String encryptedMsg = encryptFunc(message, shift); System.out.println("The Original Message: " + message); System.out.println("The Encrypted Message: " + encryptedMsg); } }
Following is the output of the above example −
Input/Output
The Original Message: Hello, Everyone there! The Encrypted Message: Khoor, Hyhubrqh wkhuh!
Features of Caesar Cipher
Caesar Cipher is one of the easy and oldest encryption method. It utilizes the technique in which we shift each letter in the plaintext by a fixed number of positions to generate the ciphertext.
It is easy to implement Caesar Cipher using basic string manipulation and modulo arithmetic operations in programming languages.
It can be easily customized by changing the shift value and it allows for different levels of encryption.
Encryption and decryption the use of Caesar Cipher are speedy and efficient, but for short messages.
As there are only 26 letters within the English alphabet, the keysize of the Caesar cipher is quite small, with best 26 possible keys.
Drawbacks of Caesar Cipher
Caesar Cipher has very weak security as it has only 26 possible keys. So this makes it easy for hackers to try all options and decrypt the messages.
The fixed alphabet size also makes it vulnerable to frequency analysis attacks, where hackers use the commonness of letters to guess the key.
Modifications to the ciphertext can also go undetected because it lacks authentication.
Because of these weaknesses, it is not suitable for modern encryption requirements.
Summary
Caesar Cipher is a simple way to hide messages. It shifts each letter in a message by using a fixed number of spaces. To use it we can choose a number for shift and move every letter by that number to encrypt the message. But it is not very secure because there are only 26 possible keys so attackers can easily guess the code. As they can use letter frequency to guess the actual letter. So with this reason it is not used nowadays.
To Continue Learning Please Login