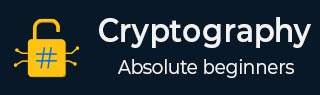
- Cryptography Tutorial
- Cryptography - Home
- Cryptography - Origin
- Cryptography - History
- Cryptography - Principles
- Cryptography - Applications
- Cryptography - Benefits & Drawbacks
- Cryptography - Modern Age
- Cryptography - Traditional Ciphers
- Cryptography - Need for Encryption
- Cryptography - Double Strength Encryption
- Cryptosystems
- Cryptosystems
- Cryptosystems - Components
- Attacks On Cryptosystem
- Cryptosystems - Rainbow table attack
- Cryptosystems - Dictionary attack
- Cryptosystems - Brute force attack
- Cryptosystems - Cryptanalysis Techniques
- Types of Cryptography
- Cryptosystems - Types
- Public Key Encryption
- Modern Symmetric Key Encryption
- Cryptography Hash functions
- Key Management
- Cryptosystems - Key Generation
- Cryptosystems - Key Storage
- Cryptosystems - Key Distribution
- Cryptosystems - Key Revocation
- Block Ciphers
- Cryptosystems - Stream Cipher
- Cryptography - Block Cipher
- Cryptography - Feistel Block Cipher
- Block Cipher Modes of Operation
- Block Cipher Modes of Operation
- Electronic Code Book (ECB) Mode
- Cipher Block Chaining (CBC) Mode
- Cipher Feedback (CFB) Mode
- Output Feedback (OFB) Mode
- Counter (CTR) Mode
- Classic Ciphers
- Cryptography - Reverse Cipher
- Cryptography - Caesar Cipher
- Cryptography - ROT13 Algorithm
- Cryptography - Transposition Cipher
- Cryptography - Encryption Transposition Cipher
- Cryptography - Decryption Transposition Cipher
- Cryptography - Multiplicative Cipher
- Cryptography - Affine Ciphers
- Cryptography - Simple Substitution Cipher
- Cryptography - Encryption of Simple Substitution Cipher
- Cryptography - Decryption of Simple Substitution Cipher
- Cryptography - Vigenere Cipher
- Cryptography - Implementing Vigenere Cipher
- Modern Ciphers
- Base64 Encoding & Decoding
- Cryptography - XOR Encryption
- Substitution techniques
- Cryptography - MonoAlphabetic Cipher
- Cryptography - Hacking Monoalphabetic Cipher
- Cryptography - Polyalphabetic Cipher
- Cryptography - Playfair Cipher
- Cryptography - Hill Cipher
- Polyalphabetic Ciphers
- Cryptography - One-Time Pad Cipher
- Implementation of One Time Pad Cipher
- Cryptography - Transposition Techniques
- Cryptography - Rail Fence Cipher
- Cryptography - Columnar Transposition
- Cryptography - Steganography
- Symmetric Algorithms
- Cryptography - Data Encryption
- Cryptography - Encryption Algorithms
- Cryptography - Data Encryption Standard
- Cryptography - Triple DES
- Cryptography - Double DES
- Advanced Encryption Standard
- Cryptography - AES Structure
- Cryptography - AES Transformation Function
- Cryptography - Substitute Bytes Transformation
- Cryptography - ShiftRows Transformation
- Cryptography - MixColumns Transformation
- Cryptography - AddRoundKey Transformation
- Cryptography - AES Key Expansion Algorithm
- Cryptography - Blowfish Algorithm
- Cryptography - SHA Algorithm
- Cryptography - RC4 Algorithm
- Cryptography - Camellia Encryption Algorithm
- Cryptography - ChaCha20 Encryption Algorithm
- Cryptography - CAST5 Encryption Algorithm
- Cryptography - SEED Encryption Algorithm
- Cryptography - SM4 Encryption Algorithm
- IDEA - International Data Encryption Algorithm
- Public Key (Asymmetric) Cryptography Algorithms
- Cryptography - RSA Algorithm
- Cryptography - RSA Encryption
- Cryptography - RSA Decryption
- Cryptography - Creating RSA Keys
- Cryptography - Hacking RSA Cipher
- Cryptography - ECDSA Algorithm
- Cryptography - DSA Algorithm
- Cryptography - Diffie-Hellman Algorithm
- Data Integrity in Cryptography
- Data Integrity in Cryptography
- Message Authentication
- Cryptography Digital signatures
- Public Key Infrastructure
- Cryptography Useful Resources
- Cryptography - Quick Guide
- Cryptography - Discussion
Cryptography - Hacking Monoalphabetic Cipher
In recent years, technology has become taken over in everyday life. It streamlines tasks like bill payment and online shopping. However, people often store sensitive information in online accounts, unaware of the ease with which hackers can access it. To understand this vulnerability, one must adopt the mindset of a hacker to recognize how they bypass security measures employed by companies.
In cybersecurity, while companies are responsible for 50% of the protection, the other half lies with users and their effective use of provided security tools. Hackers employ techniques such as Frequency Analysis, Brute Force, and Phishing. Frequency Analysis is a basic cryptanalytic method used to crack monoalphabetic ciphers.
Methods for Hacking Monoalphabetic Cipher
Here are some of the methods for hacking or cryptanalysis a monoalphabetic cipher −
Frequency Analysis − Different letters show up more often in English. The most common one is "E." Studying how often letters pop up in the coded text can help you figure out which letter might stand for "E." Once you know that, you can figure out the other letters.
Pattern Spotting − See if there are any sequences that keep coming up in the coded text. Some combos of letters, like "TH" or "ING," happen a lot in English. If you notice these combos, you can take a stab at which letters they represent.
Guess and Check − Make smart guesses based on context. Example: If you suspect a word is "THE," guess the letters that likely represent "T," "H," and "E." Use this to uncover additional letters.
Known Plaintext Attack − If you have both the original message (plaintext) and the encrypted message (ciphertext), you can use this information to figure out the encryption key. This method is very effective but often requires more resources.
Brute Force − As a last resort, try every possible combination of letters until you find the correct one. This method takes a lot of time and is usually only feasible for short messages.
Implementation using Python
A monoalphabetic cipher uses a fixed substitution to encrypt the entire message. A monoalphabetic cipher utilising a Python dictionary and JSON objects. With the use of this dictionary, we can encrypt the letters and store the corresponding letters as values in JSON. The following program creates a monoalphabetic program in the form of a class that includes all encryption and decryption functions.
Example
from string import ascii_letters, digits from random import shuffle def random_monoalpha_cipher(pool=None): if pool is None: pool = ascii_letters + digits original_pool = list(pool) shuffled_pool = list(pool) shuffle(shuffled_pool) return dict(zip(original_pool, shuffled_pool)) def inverse_monoalpha_cipher(monoalpha_cipher): inverse_monoalpha = {} for key, value in monoalpha_cipher.items(): inverse_monoalpha[value] = key return inverse_monoalpha def encrypt_with_monoalpha(message, monoalpha_cipher): encrypted_message = [] for letter in message: encrypted_message.append(monoalpha_cipher.get(letter, letter)) return ''.join(encrypted_message) def decrypt_with_monoalpha(encrypted_message, monoalpha_cipher): return encrypt_with_monoalpha( encrypted_message, inverse_monoalpha_cipher(monoalpha_cipher) ) # Generate a random monoalphabetic cipher cipher = random_monoalpha_cipher() print("Cipher:", cipher) # Encrypt a message message = 'Hello all you hackers out there!' encrypted = encrypt_with_monoalpha(message, cipher) print("Encrypted:", encrypted) # Decrypt the message decrypted = decrypt_with_monoalpha(encrypted, cipher) print("Decrypted:", decrypted)
When you implement the code given above, you will get the following output.
Input/Output
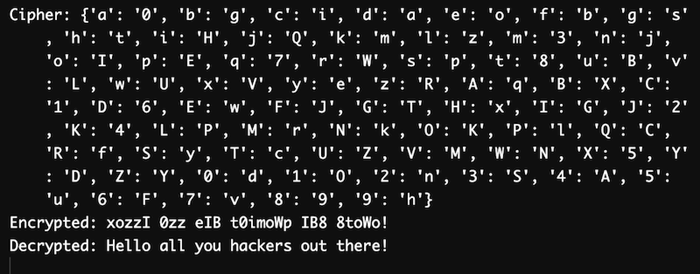
As a result, you can crack a monoalphabetic cipher using a defined key value combination, converting the ciphertext to plaintext.
Implementation using Java
The Java code is provided below has similar functionality to the Python code we have mentioned above. It can create a random monoalphabetic cipher, encrypt messages using that cipher, and decrypt the encrypted messages. See the code below −
Example
import java.util.*; public class MonoalphabeticCipher { public static Map<Character, Character> randomMonoalphaCipher(String pool) { List<Character> originalChar = new ArrayList<>(); List<Character> ShuffledChar = new ArrayList<>(); for (char c : pool.toCharArray()) { originalChar.add(c); ShuffledChar.add(c); } Collections.shuffle(ShuffledChar); Map<Character, Character> cipher = new HashMap<>(); for (int i = 0; i < originalChar.size(); i++) { cipher.put(originalChar.get(i), ShuffledChar.get(i)); } return cipher; } public static Map<Character, Character> inverseCharCipher(Map<Character, Character> monoalphaCipher) { Map<Character, Character> inverseChar = new HashMap<>(); for (Map.Entry<Character, Character> entry : monoalphaCipher.entrySet()) { inverseChar.put(entry.getValue(), entry.getKey()); } return inverseChar; } public static String encryptMessage(String message, Map<Character, Character> monoalphaCipher) { StringBuilder etMsg = new StringBuilder(); for (char letter : message.toCharArray()) { etMsg.append(monoalphaCipher.getOrDefault(letter, letter)); } return etMsg.toString(); } public static String decryptMessage(String etMsg, Map<Character, Character> monoalphaCipher) { return encryptMessage(etMsg, inverseCharCipher(monoalphaCipher)); } public static void main(String[] args) { String characters = "abcdefghijklmnopqrstuvwxyzABCDEFGHIJKLMNOPQRSTUVWXYZ0123456789"; Map<Character, Character> cipher = randomMonoalphaCipher(characters); System.out.println("Cipher: " + cipher); String message = "Hello all you hackers out there!"; String encrypted = encryptMessage(message, cipher); System.out.println("Encrypted: " + encrypted); String decrypted = decryptMessage(encrypted, cipher); System.out.println("Decrypted: " + decrypted); } }
Following is the output of the above example −
Input/Output
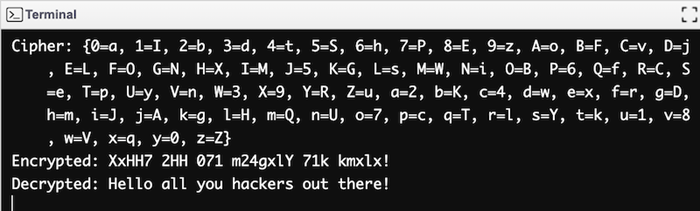
To Continue Learning Please Login