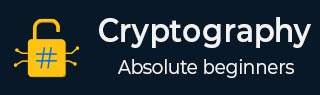
- Cryptography Tutorial
- Cryptography - Home
- Cryptography - Origin
- Cryptography - History
- Cryptography - Principles
- Cryptography - Applications
- Cryptography - Benefits & Drawbacks
- Cryptography - Modern Age
- Cryptography - Traditional Ciphers
- Cryptography - Need for Encryption
- Cryptography - Double Strength Encryption
- Cryptosystems
- Cryptosystems
- Cryptosystems - Components
- Attacks On Cryptosystem
- Cryptosystems - Rainbow table attack
- Cryptosystems - Dictionary attack
- Cryptosystems - Brute force attack
- Cryptosystems - Cryptanalysis Techniques
- Types of Cryptography
- Cryptosystems - Types
- Public Key Encryption
- Modern Symmetric Key Encryption
- Cryptography Hash functions
- Key Management
- Cryptosystems - Key Generation
- Cryptosystems - Key Storage
- Cryptosystems - Key Distribution
- Cryptosystems - Key Revocation
- Block Ciphers
- Cryptosystems - Stream Cipher
- Cryptography - Block Cipher
- Cryptography - Feistel Block Cipher
- Block Cipher Modes of Operation
- Block Cipher Modes of Operation
- Electronic Code Book (ECB) Mode
- Cipher Block Chaining (CBC) Mode
- Cipher Feedback (CFB) Mode
- Output Feedback (OFB) Mode
- Counter (CTR) Mode
- Classic Ciphers
- Cryptography - Reverse Cipher
- Cryptography - Caesar Cipher
- Cryptography - ROT13 Algorithm
- Cryptography - Transposition Cipher
- Cryptography - Encryption Transposition Cipher
- Cryptography - Decryption Transposition Cipher
- Cryptography - Multiplicative Cipher
- Cryptography - Affine Ciphers
- Cryptography - Simple Substitution Cipher
- Cryptography - Encryption of Simple Substitution Cipher
- Cryptography - Decryption of Simple Substitution Cipher
- Cryptography - Vigenere Cipher
- Cryptography - Implementing Vigenere Cipher
- Modern Ciphers
- Base64 Encoding & Decoding
- Cryptography - XOR Encryption
- Substitution techniques
- Cryptography - MonoAlphabetic Cipher
- Cryptography - Hacking Monoalphabetic Cipher
- Cryptography - Polyalphabetic Cipher
- Cryptography - Playfair Cipher
- Cryptography - Hill Cipher
- Polyalphabetic Ciphers
- Cryptography - One-Time Pad Cipher
- Implementation of One Time Pad Cipher
- Cryptography - Transposition Techniques
- Cryptography - Rail Fence Cipher
- Cryptography - Columnar Transposition
- Cryptography - Steganography
- Symmetric Algorithms
- Cryptography - Data Encryption
- Cryptography - Encryption Algorithms
- Cryptography - Data Encryption Standard
- Cryptography - Triple DES
- Cryptography - Double DES
- Advanced Encryption Standard
- Cryptography - AES Structure
- Cryptography - AES Transformation Function
- Cryptography - Substitute Bytes Transformation
- Cryptography - ShiftRows Transformation
- Cryptography - MixColumns Transformation
- Cryptography - AddRoundKey Transformation
- Cryptography - AES Key Expansion Algorithm
- Cryptography - Blowfish Algorithm
- Cryptography - SHA Algorithm
- Cryptography - RC4 Algorithm
- Cryptography - Camellia Encryption Algorithm
- Cryptography - ChaCha20 Encryption Algorithm
- Cryptography - CAST5 Encryption Algorithm
- Cryptography - SEED Encryption Algorithm
- Cryptography - SM4 Encryption Algorithm
- IDEA - International Data Encryption Algorithm
- Public Key (Asymmetric) Cryptography Algorithms
- Cryptography - RSA Algorithm
- Cryptography - RSA Encryption
- Cryptography - RSA Decryption
- Cryptography - Creating RSA Keys
- Cryptography - Hacking RSA Cipher
- Cryptography - ECDSA Algorithm
- Cryptography - DSA Algorithm
- Cryptography - Diffie-Hellman Algorithm
- Data Integrity in Cryptography
- Data Integrity in Cryptography
- Message Authentication
- Cryptography Digital signatures
- Public Key Infrastructure
- Cryptography Useful Resources
- Cryptography - Quick Guide
- Cryptography - Discussion
Cryptography - Encryption Transposition Cipher
In the previous chapter we learned about transposition cipher, types basic implementation, features and drawbacks of this technique. Now we will see the transposition cipher encryption algorithm and its implementation using different languages like Python, C++, and Java. So first let us see the transposition algorithm.
Algorithm for Transposition Cipher Encryption
A transposition cipher is a method of encrypting a message by rearranging its letters. We need a key to understand the specific order in which the characters are rearranged. The key can be a number or a keyword that is used to transposition pattern.
Here is the step by step process for encryption −
First we need to break the message into smaller units or individual characters.
Then define a grid as per the key. The number of rows is found by the length of the key, and the number of columns is by the message length divided by the number of rows.
Write the message characters row by row into the grid. If the message does not perfectly fill the grid, we can pad it with additional characters like spaces or underscores.
Lastly we can rearrange the columns as per the key. The order of columns shows the order in which we read the characters to get the ciphertext.
Example
For example, if the keyword is "SECRET", we have to rearrange the message as per the letters in "SECRET".
So we will write down our message. For example, let's use the message "HELLO WORLD".
Now, for each letter in our keyword, we will rearrange the letters in our message. So, using the keyword "SECRET", we will rearrange the letters in "HELLO WORLD" as per the order of the letters in "SECRET".
In this case, the order will be: 5, 1, 2, 4, 6, 3, 7. So, "HELLO WORLD" becomes "OLHEL LWRDO".
Lastly, our encrypted message will be "OLHEL LWRDO".
That is it! We have encrypted our message using a transposition cipher.
Implementation using Python
The encryption for transposition cipher can be implemented using different methods −
Using Python List and range() function
Using pyperclip and random modules
So let us see these methods one by one in the following sections −
Using Python List and range() function
First we will build transposition cipher encryption using Python's list and some built-in functions. So we will use list to create an empty list of encrypted messages and also we will use the range() function to generate a sequence of numbers. It will be used to iterate over the columns in the message.
Example
Below is a simple Python code for the transposition cipher encryption algorithm using list and range() function. See the program below −
def transposition_encrypt(message, key): encrypted = [''] * key for col in range(key): pointer = col while pointer < len(message): encrypted[col] += message[pointer] pointer += key return ''.join(encrypted) message = "Hello Tutorialspoint" key = 7 print("Original Message: ", message) encrypted_message = transposition_encrypt(message, key) print("Encrypted message:", encrypted_message)
Following is the output of the above example −
Input/Output
Original Message: Hello Tutorialspoint Encrypted message: Husetploolrioin atTl
In the above output you can see that the actual message was Hello Tutorialspoint and the encrypted message is Husetploolrioin atTl. In the encrypted message all the letters are the same as the original message but their orders are different.
Using pyperclip and random modules
As you may know that a random module of python is used to generate random numbers within the given range. And the pyperclip module of Python is mainly used to copy and paste clipboard functions. So in this example we are going to use these modules to generate random keys and paste the encrypted message to the clipboard. We will use random.randint() function to create a random integer key within the given range. This randomness adds a layer of unpredictability to the encryption process.
Example
Here is the implementation of a transposition cipher encryption using the random key. Check the code below −
import pyperclip import random def generate_random_key(): return random.randint(2, 10) def transposition_encrypt(message, key): encrypted = [''] * key for col in range(key): pointer = col while pointer < len(message): encrypted[col] += message[pointer] pointer += key return ''.join(encrypted) message = "Have a great day!" key = generate_random_key() print("Original Message: ", message) encrypted_message = transposition_encrypt(message, key) pyperclip.copy(encrypted_message) print("Encrypted message:", encrypted_message) print("Key used for encryption:", key) print("Encrypted message copied to clipboard!")
Following is the output of the above example −
Input/Output
Original Message: Have a great day! Encrypted message: Heaavte daa yg!r Key used for encryption: 9 Encrypted message copied to clipboard!
Original Message: Have a great day! Encrypted message: H r !aaedv aaegty Key used for encryption: 4 Encrypted message copied to clipboard!
In the above output you can notice that a random key is used every time to generate an encrypted message.
Implementation using Java
In this we are going to use Java programming language to implment the encryption transposition cipher. A plaintext string and a key are needed as input parameters for the encrypt method. According to the length of the plaintext and the key, it defines how many rows are needed. Next, it rows-by-row generates a matrix with the plaintext characters. After that, the ciphertext is created by reading the matrix column by column. Then we can use the main the encrypt method in the main method.
Example
Below is the implementation of the encryption transposition cipher using Java programming langugage −
public class TranspositionCipher { // Function to encrypt using transposition cipher public static String encrypt(String plaintext, int key) { StringBuilder ciphertext = new StringBuilder(); int rows = (int) Math.ceil((double) plaintext.length() / key); char[][] matrix = new char[rows][key]; // Fill matrix with plaintext characters int index = 0; for (int i = 0; i < rows; ++i) { for (int j = 0; j < key; ++j) { if (index < plaintext.length()) matrix[i][j] = plaintext.charAt(index++); else matrix[i][j] = ' '; } } // Read matrix column-wise to get ciphertext for (int j = 0; j < key; ++j) { for (int i = 0; i < rows; ++i) { ciphertext.append(matrix[i][j]); } } return ciphertext.toString(); } public static void main(String[] args) { String plaintext = "Hello, This is very beautiful world"; int key = 4; System.out.println("The Plaintext Message is: " + plaintext); String encryptedText = encrypt(plaintext, key); System.out.println("The Encrypted text: " + encryptedText); } }
Following is the output of the above example −
Input/Output
The Plaintext Message is: Hello, This is very beautiful world The Encrypted text: Hohiebtlre,isrei ll s yafwdlT v uuo
Implementation using C++
Now we will use C++ to implment the encryption of transposition cipher. A plaintext string and a key are the input parameters for the encrypt function. According to the length of the plaintext and the key, it find how many rows are needed. Next, it rows-by-row populates a matrix with the plaintext characters. After that, the ciphertext is constructed by reading the matrix column by column. The encrypt function's usage is seen in the main function.
Example
See the below implementation using C++ for encryption transposition cipher −
#include <iostream> #include <string> #include <algorithm> // Function to encrypt using transposition cipher std::string encrypt(std::string plaintext, int key) { std::string ciphertext = ""; int rows = (plaintext.length() + key - 1) / key; char matrix[rows][key]; // Fill matrix with plaintext characters int index = 0; for (int i = 0; i < rows; ++i) { for (int j = 0; j < key; ++j) { if (index < plaintext.length()) matrix[i][j] = plaintext[index++]; else matrix[i][j] = ' '; } } // Read matrix column-wise to get ciphertext for (int j = 0; j < key; ++j) { for (int i = 0; i < rows; ++i) { ciphertext += matrix[i][j]; } } return ciphertext; } int main() { std::string plaintext = "Hello, Tutorialspoint Family"; int key = 4; std::cout << "The Plaintext message is: " << plaintext << std::endl; std::string encrypted_msg = encrypt(plaintext, key); std::cout << "The Encrypted text: " << encrypted_msg << std::endl; return 0; }
Following is the output of the above example −
Input/Output
The Plaintext message is: Hello, Tutorialspoint Family The Encrypted text: Houiptme,tao il oliFllTrsnay
Summary
A straightforward encryption method called the Transposition Cipher rearranges a message's characters according to the given key. We looked at a number of Python implementations of the Transposition Cipher encryption technique in this chapter. These implementations included using Python lists and range functions, Pyperclip module for clipboard functionality, Columnar Order method, and generating random keys for encryption.
To Continue Learning Please Login