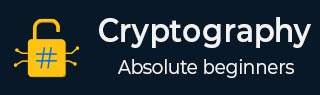
- Cryptography Tutorial
- Cryptography - Home
- Cryptography - Origin
- Cryptography - History
- Cryptography - Principles
- Cryptography - Applications
- Cryptography - Benefits & Drawbacks
- Cryptography - Modern Age
- Cryptography - Traditional Ciphers
- Cryptography - Need for Encryption
- Cryptography - Double Strength Encryption
- Cryptosystems
- Cryptosystems
- Cryptosystems - Components
- Attacks On Cryptosystem
- Cryptosystems - Rainbow table attack
- Cryptosystems - Dictionary attack
- Cryptosystems - Brute force attack
- Cryptosystems - Cryptanalysis Techniques
- Types of Cryptography
- Cryptosystems - Types
- Public Key Encryption
- Modern Symmetric Key Encryption
- Cryptography Hash functions
- Key Management
- Cryptosystems - Key Generation
- Cryptosystems - Key Storage
- Cryptosystems - Key Distribution
- Cryptosystems - Key Revocation
- Block Ciphers
- Cryptosystems - Stream Cipher
- Cryptography - Block Cipher
- Cryptography - Feistel Block Cipher
- Block Cipher Modes of Operation
- Block Cipher Modes of Operation
- Electronic Code Book (ECB) Mode
- Cipher Block Chaining (CBC) Mode
- Cipher Feedback (CFB) Mode
- Output Feedback (OFB) Mode
- Counter (CTR) Mode
- Classic Ciphers
- Cryptography - Reverse Cipher
- Cryptography - Caesar Cipher
- Cryptography - ROT13 Algorithm
- Cryptography - Transposition Cipher
- Cryptography - Encryption Transposition Cipher
- Cryptography - Decryption Transposition Cipher
- Cryptography - Multiplicative Cipher
- Cryptography - Affine Ciphers
- Cryptography - Simple Substitution Cipher
- Cryptography - Encryption of Simple Substitution Cipher
- Cryptography - Decryption of Simple Substitution Cipher
- Cryptography - Vigenere Cipher
- Cryptography - Implementing Vigenere Cipher
- Modern Ciphers
- Base64 Encoding & Decoding
- Cryptography - XOR Encryption
- Substitution techniques
- Cryptography - MonoAlphabetic Cipher
- Cryptography - Hacking Monoalphabetic Cipher
- Cryptography - Polyalphabetic Cipher
- Cryptography - Playfair Cipher
- Cryptography - Hill Cipher
- Polyalphabetic Ciphers
- Cryptography - One-Time Pad Cipher
- Implementation of One Time Pad Cipher
- Cryptography - Transposition Techniques
- Cryptography - Rail Fence Cipher
- Cryptography - Columnar Transposition
- Cryptography - Steganography
- Symmetric Algorithms
- Cryptography - Data Encryption
- Cryptography - Encryption Algorithms
- Cryptography - Data Encryption Standard
- Cryptography - Triple DES
- Cryptography - Double DES
- Advanced Encryption Standard
- Cryptography - AES Structure
- Cryptography - AES Transformation Function
- Cryptography - Substitute Bytes Transformation
- Cryptography - ShiftRows Transformation
- Cryptography - MixColumns Transformation
- Cryptography - AddRoundKey Transformation
- Cryptography - AES Key Expansion Algorithm
- Cryptography - Blowfish Algorithm
- Cryptography - SHA Algorithm
- Cryptography - RC4 Algorithm
- Cryptography - Camellia Encryption Algorithm
- Cryptography - ChaCha20 Encryption Algorithm
- Cryptography - CAST5 Encryption Algorithm
- Cryptography - SEED Encryption Algorithm
- Cryptography - SM4 Encryption Algorithm
- IDEA - International Data Encryption Algorithm
- Public Key (Asymmetric) Cryptography Algorithms
- Cryptography - RSA Algorithm
- Cryptography - RSA Encryption
- Cryptography - RSA Decryption
- Cryptography - Creating RSA Keys
- Cryptography - Hacking RSA Cipher
- Cryptography - ECDSA Algorithm
- Cryptography - DSA Algorithm
- Cryptography - Diffie-Hellman Algorithm
- Data Integrity in Cryptography
- Data Integrity in Cryptography
- Message Authentication
- Cryptography Digital signatures
- Public Key Infrastructure
- Cryptography Useful Resources
- Cryptography - Quick Guide
- Cryptography - Discussion
Cryptography - Implementing Vigenere Cipher
In the last chapter we saw Vigenere Cipher, its methods, strengths weeknesses. Now we will implement vigenere cipher using different programming langugages like Python, Java, and C++.
Implementation using Python
The Vigenere cipher, a technique for encrypting and decrypting text messages, is implemented in this Python code. The generate_key() function takes a keyword as input, creates a key based on that keyword, then repeats the words as needed to make sure the key fits the text length. The Vigenere cipher algorithm, which shifts each character according to its matching character in the key, is used by the encrypt_text() function to encrypt the text. In the same way, by reversing the encryption process, the decrypt_text() method decrypts the ciphertext and shows the original text.
Example
Following is the implementation of Vigenere Cipher with the help of Python −
def generate_key(text, keyword): key = list(keyword) if len(text) == len(keyword): return key else: for i in range(len(text) - len(keyword)): key.append(key[i % len(keyword)]) return "".join(key) def encrypt_text(text, key): cipher_text = [] for i in range(len(text)): x = (ord(text[i]) + ord(key[i])) % 26 x += ord('A') cipher_text.append(chr(x)) return "".join(cipher_text) def decrypt_text(cipher_text, key): original_text = [] for i in range(len(cipher_text)): x = (ord(cipher_text[i]) - ord(key[i]) + 26) % 26 x += ord('A') original_text.append(chr(x)) return "".join(original_text) # Driver code text = "TUTORIALSPOINT" keyword = "KEY" key = generate_key(text, keyword) cipher_text = encrypt_text(text, key) print("The Encrypted text:", cipher_text) print("The Original/Decrypted Text:", decrypt_text(cipher_text, key))
Following is the output of the above example −
Input/Output
The Encrypted text: DYRYVGKPQZSGXX The Original/Decrypted Text: TUTORIALSPOINT
Implementation using Java
A method used for encrypting and decrypting messages is the Vigenere Cipher. The length of the text message and a keyword are used in the code to generate a key. The text message is then encrypted with the help of this key by shifting each character in the alphabet to match its proper location in the key. The same key is used to reverse the encryption process and return each character to its original alphabetic position in order to decrypt the message. With the original message as the result, the parties that have the key can communicate privately with one another.
Example
See the Java implementation below for vigenere cipher −
public class VigenereCipher { static String generateKey(String text, String keyword) { int textLength = text.length(); for (int i = 0; ; i++) { if (textLength == i) i = 0; if (keyword.length() == textLength) break; keyword += (keyword.charAt(i)); } return keyword; } static String encryptText(String text, String keyword) { String ciphertext = ""; for (int i = 0; i < text.length(); i++) { // converting in range 0-25 int x = (text.charAt(i) + keyword.charAt(i)) % 26; // convert into alphabets(ASCII) x += 'A'; ciphertext += (char)(x); } return ciphertext; } // Decrypt the encrypted text and return the original text static String decryptText(String ciphertext, String keyword) { String originalText = ""; for (int i = 0 ; i < ciphertext.length() && i < keyword.length(); i++) { // converting in range 0-25 int x = (ciphertext.charAt(i) - keyword.charAt(i) + 26) % 26; // convert into alphabets(ASCII) x += 'A'; originalText += (char)(x); } return originalText; } // Driver code public static void main(String[] args) { String message = "Hello everyone"; String keyword = "Best"; String text = message.toUpperCase(); String key = keyword.toUpperCase(); String generatedKey = generateKey(text, key); String encryptedText = encryptText(text, generatedKey); System.out.println("The Encrypted Text : " + encryptedText); System.out.println("The Original/Decrypted Text : " + decryptText(encryptedText, generatedKey)); } }
Following is the output of the above example −
Input/Output
The Encrypted Text : IIDEPXWOFVQHOI The Original/Decrypted Text : HELLOTEVERYONE
Implementation using C++
We will use the C++ programming language to implement the Vigenere Cypher in this code. We will apply a set of Caesar shifts to the plaintext message's characters in this cipher using a key. Each letter in the key relates to a distinct shift value, and the key defines the amount of shift applied to each character. Every character in the plaintext is shifted forward in the alphabet in encryption by the matching number given by the key.
In a similar way, the original plaintext becomes visible in decryption by shifting the ciphertext's characters backward by the same amount shown in the key.
Example
Below is the simple implementation for vigenere cipher using C++ programming language −
#include <iostream> #include <string> using namespace std; class VigenereCipher { public: string key; VigenereCipher(string key) { for (int i = 0; i < key.size(); ++i) { if (key[i] >= 'A' && key[i] <= 'Z') this -> key += key[i]; else if (key[i] >= 'a' && key[i] <= 'z') this -> key += key[i] + 'A' - 'a'; } } string encryptFunc(string text) { string out; for (int i = 0, j = 0; i < text.length(); ++i) { char c = text[i]; if (c >= 'a' && c <= 'z') c += 'A' - 'a'; else if (c < 'A' || c > 'Z') continue; out += (c + key[j] - 2 * 'A') % 26 + 'A'; j = (j + 1) % key.length(); } return out; } string decryptFunc(string text) { string out; for (int i = 0, j = 0; i < text.length(); ++i) { char c = text[i]; if (c >= 'a' && c <= 'z') c += 'A' - 'a'; else if (c < 'A' || c > 'Z') continue; out += (c - key[j] + 26) % 26 + 'A'; j = (j + 1) % key.length(); } return out; } }; int main() { VigenereCipher cipher("VigenereCipher"); string plaintext = "Cyber Security"; string et = cipher.encryptFunc(plaintext); string dt = cipher.decryptFunc(et); cout << "The Plaintext: " << plaintext << endl; cout << "The Encrypted Text: " << et << endl; cout << "The Decrypted Text: " << dt << endl; }
Following is the output of the above example −
Input/Output
The Plaintext: Cyber Security The Encrypted Text: XGHIEWVGWZXAC The Decrypted Text: CYBERSECURITY
To Continue Learning Please Login