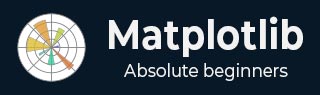
- Matplotlib Basics
- Matplotlib - Home
- Matplotlib - Introduction
- Matplotlib - Vs Seaborn
- Matplotlib - Environment Setup
- Matplotlib - Anaconda distribution
- Matplotlib - Jupyter Notebook
- Matplotlib - Pyplot API
- Matplotlib - Simple Plot
- Matplotlib - Saving Figures
- Matplotlib - Markers
- Matplotlib - Figures
- Matplotlib - Styles
- Matplotlib - Legends
- Matplotlib - Colors
- Matplotlib - Colormaps
- Matplotlib - Colormap Normalization
- Matplotlib - Choosing Colormaps
- Matplotlib - Colorbars
- Matplotlib - Text
- Matplotlib - Text properties
- Matplotlib - Subplot Titles
- Matplotlib - Images
- Matplotlib - Image Masking
- Matplotlib - Annotations
- Matplotlib - Arrows
- Matplotlib - Fonts
- Matplotlib - What are Fonts?
- Setting Font Properties Globally
- Matplotlib - Font Indexing
- Matplotlib - Mathematical Expressions
- Matplotlib - Transforms
- Matplotlib - Ticks and Tick Labels
- Matplotlib - Axis Ranges
- Matplotlib - Axis Scales
- Matplotlib - Axis Ticks
- Matplotlib - Formatting Axes
- Matplotlib - Axes Class
- Matplotlib - Twin Axes
- Matplotlib - Figure Class
- Matplotlib - Multiplots
- Matplotlib - Grids
- Matplotlib - Object-oriented Interface
- Matplotlib - PyLab module
- Matplotlib - Subplots() Function
- Matplotlib - Subplot2grid() Function
- Matplotlib - Anchored Artists
- Matplotlib - Manual Contour
- Matplotlib - Coords Report
- Matplotlib - AGG filter
- Matplotlib - Ribbon Box
- Matplotlib - Fill Spiral
- Matplotlib - Findobj Demo
- Matplotlib - Hyperlinks
- Matplotlib - Image Thumbnail
- Matplotlib - Plotting with Keywords
- Matplotlib - Create Logo
- Matplotlib - Multipage PDF
- Matplotlib - Multiprocessing
- Matplotlib - Print Stdout
- Matplotlib - Compound Path
- Matplotlib - Sankey Class
- Matplotlib - MRI with EEG
- Matplotlib - Stylesheets
- Matplotlib - Background Colors
- Matplotlib - Basemap
- Matplotlib Event Handling
- Matplotlib - Event Handling
- Matplotlib - Close Event
- Matplotlib - Mouse Move
- Matplotlib - Click Events
- Matplotlib - Scroll Event
- Matplotlib - Keypress Event
- Matplotlib - Pick Event
- Matplotlib - Looking Glass
- Matplotlib - Path Editor
- Matplotlib - Poly Editor
- Matplotlib - Timers
- Matplotlib - Viewlims
- Matplotlib - Zoom Window
- Matplotlib Plotting
- Matplotlib - Bar Graphs
- Matplotlib - Histogram
- Matplotlib - Pie Chart
- Matplotlib - Scatter Plot
- Matplotlib - Box Plot
- Matplotlib - Violin Plot
- Matplotlib - Contour Plot
- Matplotlib - 3D Plotting
- Matplotlib - 3D Contours
- Matplotlib - 3D Wireframe Plot
- Matplotlib - 3D Surface Plot
- Matplotlib - Quiver Plot
- Matplotlib Useful Resources
- Matplotlib - Quick Guide
- Matplotlib - Useful Resources
- Matplotlib - Discussion
Matplotlib - Multipage PDF
A multipage PDF(Portable Document Format) is a type of file that can store multiple pages or images in a single document. Each page within the PDF can have different content, such as plots, images, or text.
Matplotlib provides support for creating multipage PDFs through its backend_pdf.PdfPages module. This feature allows users to save plots and visualizations across multiple pages within the same PDF file.
In certain situations, it becomes necessary to save several plots in one file. While many image file formats like PNG, SVG, or JPEG typically support only a single image per file, Matplotlib provides a solution for creating multipage output. PDF is one such supported format, allowing users to organize and share visualizations effectively.
Creating a Basic multipage PDF
To save plots on multiple pages in a PDF document using Matplotlib, you can make use of the PdfPages class. This class simplifies the process of generating a PDF file with several pages, each containing different visualizations.
Example
Let's start with a basic example demonstrating how to create a multipage PDF with Matplotlib. This example saves multiple figures in one PDF file at once.
from matplotlib.backends.backend_pdf import PdfPages import numpy as np import matplotlib.pyplot as plt # sample data for plots x1 = np.arange(10) y1 = x1**2 x2 = np.arange(20) y2 = x2**2 # Create a PdfPages object to save the pages pp = PdfPages('Basic_multipage_pdf.pdf') def function_plot(X,Y): plt.figure() plt.clf() plt.plot(X,Y) plt.title('y vs x') plt.xlabel('x axis', fontsize = 13) plt.ylabel('y axis', fontsize = 13) pp.savefig() # Create and save the first plot function_plot(x1,y1) # Create and save the second plot function_plot(x2,y2) pp.close()
Output
On executing the above program, 'Basic_multipage_pdf.pdf' will be generated in the directory where the script is saved.
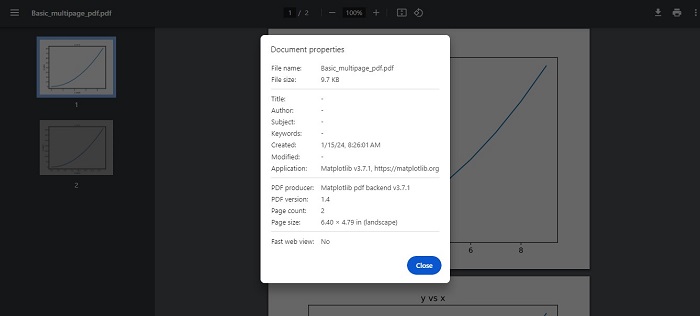
Adding Metadata and Annotations
Matplotlib also supports the addition of metadata and annotations to a multipage PDF. Metadata can include information such as the title, author, and creation date, providing additional context or details about the content within the PDF.
Example
Here is an advanced example, that creates a multipage PDF with metadata and annotations.
import datetime import matplotlib.pyplot as plt import numpy as np from matplotlib.backends.backend_pdf import PdfPages # Create the PdfPages object to save the pages with PdfPages('Advanced_multipage_pdf.pdf') as pdf: # Page One plt.figure(figsize=(3, 3)) plt.plot(range(7), [3, 1, 4, 1, 5, 9, 2], 'r-o') plt.title('Page One') # saves the current figure into a pdf page pdf.savefig() plt.close() # Page Two # Initially set it to True. If LaTeX is not installed or an error is caught, change to `False` # The usetex setting is particularly useful when you need LaTeX features that aren't present in matplotlib's built-in mathtext. plt.rcParams['text.usetex'] = False plt.figure(figsize=(8, 6)) x = np.arange(0, 5, 0.1) plt.plot(x, np.sin(x), 'b-') plt.title('Page Two') # attach metadata (as pdf note) to page pdf.attach_note("plot of sin(x)") pdf.savefig() plt.close() # Page Three plt.rcParams['text.usetex'] = False fig = plt.figure(figsize=(4, 5)) plt.plot(x, x ** 2, 'ko') plt.title('Page Three') pdf.savefig(fig) plt.close() # Set file metadata d = pdf.infodict() d['Title'] = 'Multipage PDF Example' d['Author'] = 'Tutorialspoint' d['Subject'] = 'How to create a multipage pdf file and set its metadata' d['Keywords'] = 'PdfPages multipage keywords author title subject' d['CreationDate'] = datetime.datetime(2024, 1, 15) d['ModDate'] = datetime.datetime.today()
Output
On executing the above program, 'Advanced_multipage_pdf.pdf' will be generated in the directory where the script is saved. you will be able to observe the details like below −
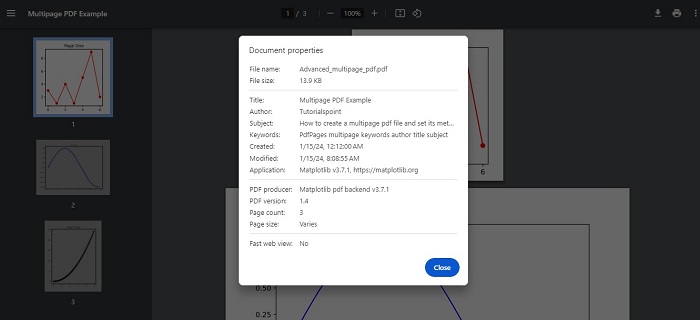
To Continue Learning Please Login