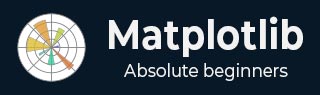
- Matplotlib Basics
- Matplotlib - Home
- Matplotlib - Introduction
- Matplotlib - Vs Seaborn
- Matplotlib - Environment Setup
- Matplotlib - Anaconda distribution
- Matplotlib - Jupyter Notebook
- Matplotlib - Pyplot API
- Matplotlib - Simple Plot
- Matplotlib - Saving Figures
- Matplotlib - Markers
- Matplotlib - Figures
- Matplotlib - Styles
- Matplotlib - Legends
- Matplotlib - Colors
- Matplotlib - Colormaps
- Matplotlib - Colormap Normalization
- Matplotlib - Choosing Colormaps
- Matplotlib - Colorbars
- Matplotlib - Text
- Matplotlib - Text properties
- Matplotlib - Subplot Titles
- Matplotlib - Images
- Matplotlib - Image Masking
- Matplotlib - Annotations
- Matplotlib - Arrows
- Matplotlib - Fonts
- Matplotlib - What are Fonts?
- Setting Font Properties Globally
- Matplotlib - Font Indexing
- Matplotlib - Mathematical Expressions
- Matplotlib - Transforms
- Matplotlib - Ticks and Tick Labels
- Matplotlib - Axis Ranges
- Matplotlib - Axis Scales
- Matplotlib - Axis Ticks
- Matplotlib - Formatting Axes
- Matplotlib - Axes Class
- Matplotlib - Twin Axes
- Matplotlib - Figure Class
- Matplotlib - Multiplots
- Matplotlib - Grids
- Matplotlib - Object-oriented Interface
- Matplotlib - PyLab module
- Matplotlib - Subplots() Function
- Matplotlib - Subplot2grid() Function
- Matplotlib - Anchored Artists
- Matplotlib - Manual Contour
- Matplotlib - Coords Report
- Matplotlib - AGG filter
- Matplotlib - Ribbon Box
- Matplotlib - Fill Spiral
- Matplotlib - Findobj Demo
- Matplotlib - Hyperlinks
- Matplotlib - Image Thumbnail
- Matplotlib - Plotting with Keywords
- Matplotlib - Create Logo
- Matplotlib - Multipage PDF
- Matplotlib - Multiprocessing
- Matplotlib - Print Stdout
- Matplotlib - Compound Path
- Matplotlib - Sankey Class
- Matplotlib - MRI with EEG
- Matplotlib - Stylesheets
- Matplotlib - Background Colors
- Matplotlib - Basemap
- Matplotlib Event Handling
- Matplotlib - Event Handling
- Matplotlib - Close Event
- Matplotlib - Mouse Move
- Matplotlib - Click Events
- Matplotlib - Scroll Event
- Matplotlib - Keypress Event
- Matplotlib - Pick Event
- Matplotlib - Looking Glass
- Matplotlib - Path Editor
- Matplotlib - Poly Editor
- Matplotlib - Timers
- Matplotlib - Viewlims
- Matplotlib - Zoom Window
- Matplotlib Plotting
- Matplotlib - Bar Graphs
- Matplotlib - Histogram
- Matplotlib - Pie Chart
- Matplotlib - Scatter Plot
- Matplotlib - Box Plot
- Matplotlib - Violin Plot
- Matplotlib - Contour Plot
- Matplotlib - 3D Plotting
- Matplotlib - 3D Contours
- Matplotlib - 3D Wireframe Plot
- Matplotlib - 3D Surface Plot
- Matplotlib - Quiver Plot
- Matplotlib Useful Resources
- Matplotlib - Quick Guide
- Matplotlib - Useful Resources
- Matplotlib - Discussion
Matplotlib - Radio Buttons
Introduction
Matplotlib provides the RadioButtons widget in the matplotlib.widgets module for creating radio button groups in plots. Radio buttons are commonly used in graphical user interfaces to allow users to choose one option from a set of mutually exclusive options. In Matplotlib library RadioButtons offer a way to integrate this interactive element directly into a plot.
Now we will explore the key features, implementation detail and use cases of RadioButtons in Matplotlib library.
Key Features
The following are the key features of the Radiobuttons widget of the matplotlib library.
Mutually Exclusive Options − RadioButtons allow users to choose only one option from a set of mutually exclusive options. When one radio button is selected the others in the group are automatically deselected.
Integration with Callback Functions − The widget is often used in conjunction with callback functions. These functions are executed when a radio button is clicked by allowing developers to define custom actions based on the user's selection.
Customizable Appearance − RadioButtons provide customization options for appearance such as the label text, color and layout. This allows developers to align the appearance of radio buttons with the overall design of the plot.
Implementation of the Radiobuttons on a plot
Let's walk through a basic example to illustrate the implementation of RadioButtons in Matplotlib library. In this example we'll create a simple plot with two radio buttons and the plot will be updated based on the selected option.
The following is the explaination of the important functions and modules used in the below example.
plot_function − This is a simple function that generates random data around a sine wave. The line style of this function will be modified based on the selected radio button.
radio_callback − This function is called when a radio button is clicked. It sets the line style of the plotted function based on the selected option and redraws the plot.
RadioButtons(rax, ('solid', 'dashed', 'dashdot', 'dotted'), active=0) − This line creates the RadioButtons widget specifying the axes (rax), the labels for the line styles, and the initially selected line style (index 0).
radio_buttons.on_clicked(radio_callback) − This line connects the on_clicked event of the RadioButtons to the radio_callback function. The radio_callback function is called when a radio button is clicked.
Example
import matplotlib.pyplot as plt from matplotlib.widgets import RadioButtons import numpy as np # Function to be triggered by radio button selection def radio_callback(label): ax.clear() # Clear the axes for updating the plot ax.plot(x, plot_function(x, label), label=label, linestyle=label) ax.legend() plt.draw() # Redraw the plot # Function to generate random data around a sine wave def plot_function(x, label): return np.sin(x) + np.random.normal(scale=0.1, size=x.shape) # Create a figure and axes fig, ax = plt.subplots() # Generate x values x = np.linspace(0, 2 * np.pi, 100) # Create RadioButtons radio_ax = plt.axes([0.05, 0.5, 0.15, 0.15]) radio_buttons = RadioButtons(radio_ax, ('solid', 'dashed', 'dashdot', 'dotted'), active=0) # Connect the radio buttons to the callback function radio_buttons.on_clicked(radio_callback) # Initialize the plot with the initial line style radio_callback('solid') plt.show()
Output
When Solid radio button selected
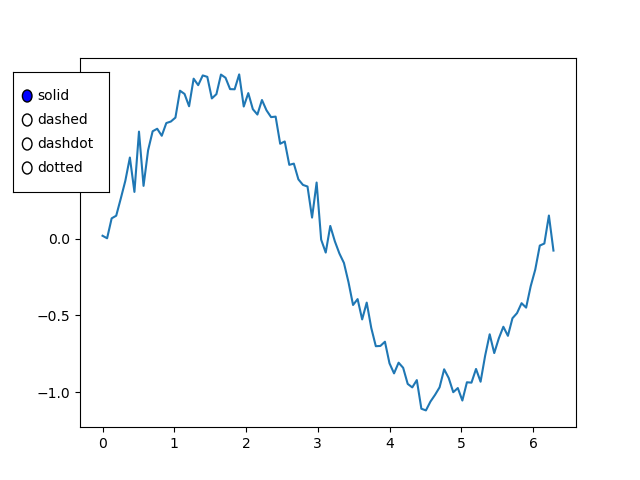
When dashed radio button selected
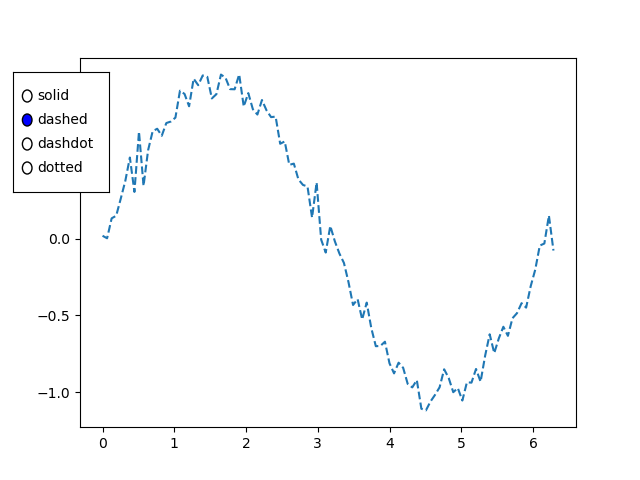
When dashdot radio button selected
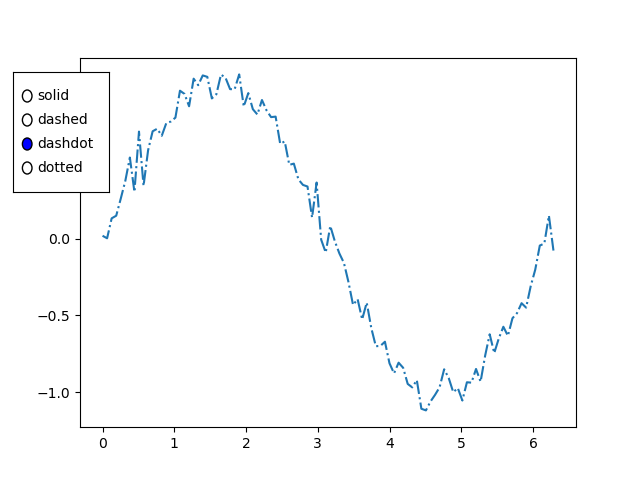
When dotted radio button selected
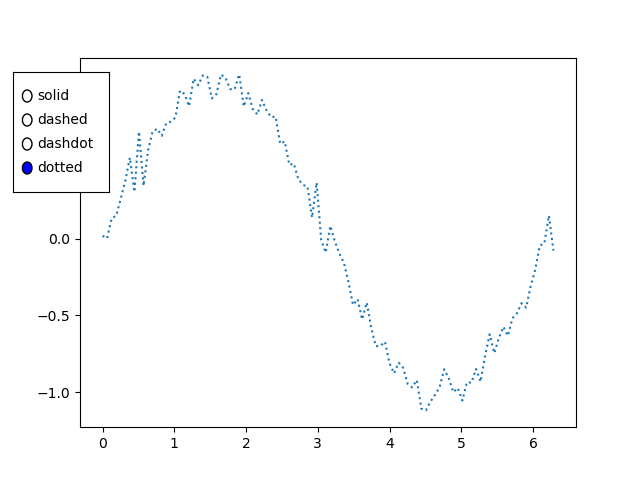
Use Cases
The below are the use cases of the radio buttons widget of the matplotli library.
Data Filtering − Radio buttons can be used to filter and display different subsets of data in a plot. Each option corresponds to a specific set of data and selecting an option updates the plot accordingly.
Parameter Selection − Radio buttons are useful for selecting different parameters or configurations in a visualization. For example we might use radio buttons to toggle between linear and logarithmic scales.
Interactive Control − Incorporating radio buttons adds interactive control to the plot by allowing users to dynamically change settings or switch between different views.
Scenario-based Visualization − In scenarios where the data can be presented in different ways such as different chart types or representations, radio buttons help users quickly switch between options.
Customization
Label and Color Customization
We can customize the labels and colors of the radio buttons to match the design of our plot. This can be achieved by providing appropriate arguments when creating the RadioButtons widget.
Layout Customization
Adjusting the layout of radio buttons as demonstrated in the example which allows us to control their position within the plot area.
To Continue Learning Please Login